mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-11-08 09:26:42 +00:00
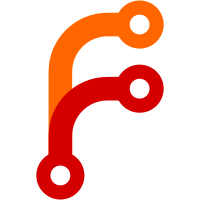
Since management of API tokens can be accessed via two routes, this adds tracking and handling to reutrn the user to the correct place.
64 lines
1.4 KiB
PHP
64 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Api;
|
|
|
|
use BookStack\Activity\Models\Loggable;
|
|
use BookStack\Users\Models\User;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsTo;
|
|
use Illuminate\Support\Carbon;
|
|
|
|
/**
|
|
* Class ApiToken.
|
|
*
|
|
* @property int $id
|
|
* @property string $token_id
|
|
* @property string $secret
|
|
* @property string $name
|
|
* @property Carbon $expires_at
|
|
* @property User $user
|
|
*/
|
|
class ApiToken extends Model implements Loggable
|
|
{
|
|
use HasFactory;
|
|
|
|
protected $fillable = ['name', 'expires_at'];
|
|
protected $casts = [
|
|
'expires_at' => 'date:Y-m-d',
|
|
];
|
|
|
|
/**
|
|
* Get the user that this token belongs to.
|
|
*/
|
|
public function user(): BelongsTo
|
|
{
|
|
return $this->belongsTo(User::class);
|
|
}
|
|
|
|
/**
|
|
* Get the default expiry value for an API token.
|
|
* Set to 100 years from now.
|
|
*/
|
|
public static function defaultExpiry(): string
|
|
{
|
|
return Carbon::now()->addYears(100)->format('Y-m-d');
|
|
}
|
|
|
|
/**
|
|
* {@inheritdoc}
|
|
*/
|
|
public function logDescriptor(): string
|
|
{
|
|
return "({$this->id}) {$this->name}; User: {$this->user->logDescriptor()}";
|
|
}
|
|
|
|
/**
|
|
* Get the URL for managing this token.
|
|
*/
|
|
public function getUrl(string $path = ''): string
|
|
{
|
|
return url("/api-tokens/{$this->user_id}/{$this->id}/" . trim($path, '/'));
|
|
}
|
|
}
|