mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-09-19 11:08:11 +00:00
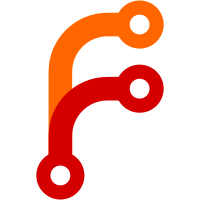
During review of #4560. - Simplified command to share as much log as possible across different run options. - Extracted out user handling to share with MFA command. - Added specific handling for disabled avatar fetching. - Added mention of avatar endpoint, to make it clear where these avatars are coming from (Protect against user expectation of LDAP avatar sync). - Simplified a range of the testing. - Tweaked wording and code formatting.
41 lines
974 B
PHP
41 lines
974 B
PHP
<?php
|
|
|
|
namespace BookStack\Console\Commands;
|
|
|
|
use BookStack\Users\Models\User;
|
|
use Exception;
|
|
use Illuminate\Console\Command;
|
|
|
|
/**
|
|
* @mixin Command
|
|
*/
|
|
trait HandlesSingleUser
|
|
{
|
|
/**
|
|
* Fetch a user provided to this command.
|
|
* Expects the command to accept 'id' and 'email' options.
|
|
* @throws Exception
|
|
*/
|
|
private function fetchProvidedUser(): User
|
|
{
|
|
$id = $this->option('id');
|
|
$email = $this->option('email');
|
|
if (!$id && !$email) {
|
|
throw new Exception("Either a --id=<number> or --email=<email> option must be provided.\nRun this command with `--help` to show more options.");
|
|
}
|
|
|
|
$field = $id ? 'id' : 'email';
|
|
$value = $id ?: $email;
|
|
|
|
$user = User::query()
|
|
->where($field, '=', $value)
|
|
->first();
|
|
|
|
if (!$user) {
|
|
throw new Exception("A user where {$field}={$value} could not be found.");
|
|
}
|
|
|
|
return $user;
|
|
}
|
|
}
|