mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-09-19 11:08:11 +00:00
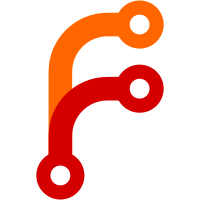
Started basic playground for testing lexical as a new WYSIWYG editor. Moved out tinymce to be under wysiwyg-tinymce instead so lexical is the default, but TinyMce code remains.
44 lines
1.3 KiB
JavaScript
44 lines
1.3 KiB
JavaScript
/**
|
|
* Setup a serializer filter for <br> tags to ensure they're not rendered
|
|
* within code blocks and that we use newlines there instead.
|
|
* @param {Editor} editor
|
|
*/
|
|
function setupBrFilter(editor) {
|
|
editor.serializer.addNodeFilter('br', nodes => {
|
|
for (const node of nodes) {
|
|
if (node.parent && node.parent.name === 'code') {
|
|
const newline = window.tinymce.html.Node.create('#text');
|
|
newline.value = '\n';
|
|
node.replace(newline);
|
|
}
|
|
}
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Remove accidentally added pointer elements that are within the content.
|
|
* These could have accidentally been added via getting caught in range
|
|
* selection within page content.
|
|
* @param {Editor} editor
|
|
*/
|
|
function setupPointerFilter(editor) {
|
|
editor.parser.addNodeFilter('div', nodes => {
|
|
for (const node of nodes) {
|
|
const id = node.attr('id') || '';
|
|
const nodeClass = node.attr('class') || '';
|
|
if (id === 'pointer' || nodeClass.includes('pointer')) {
|
|
node.remove();
|
|
}
|
|
}
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Setup global default filters for the given editor instance.
|
|
* @param {Editor} editor
|
|
*/
|
|
export function setupFilters(editor) {
|
|
setupBrFilter(editor);
|
|
setupPointerFilter(editor);
|
|
}
|