mirror of
https://github.com/BookStackApp/BookStack.git
synced 2025-01-10 02:57:36 +00:00
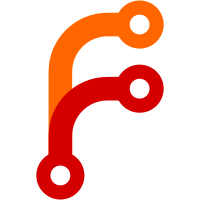
Failed notification sends could block the user action, whereas it's probably more important that the user action takes places uninteruupted than showing an error screen for the user to debug. Logs notification errors so issues can still be debugged by admins. Closes #5315
47 lines
1.7 KiB
PHP
47 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Activity\Notifications\Handlers;
|
|
|
|
use BookStack\Activity\Models\Loggable;
|
|
use BookStack\Activity\Notifications\Messages\BaseActivityNotification;
|
|
use BookStack\Entities\Models\Entity;
|
|
use BookStack\Permissions\PermissionApplicator;
|
|
use BookStack\Users\Models\User;
|
|
use Illuminate\Support\Facades\Log;
|
|
|
|
abstract class BaseNotificationHandler implements NotificationHandler
|
|
{
|
|
/**
|
|
* @param class-string<BaseActivityNotification> $notification
|
|
* @param int[] $userIds
|
|
*/
|
|
protected function sendNotificationToUserIds(string $notification, array $userIds, User $initiator, string|Loggable $detail, Entity $relatedModel): void
|
|
{
|
|
$users = User::query()->whereIn('id', array_unique($userIds))->get();
|
|
|
|
foreach ($users as $user) {
|
|
// Prevent sending to the user that initiated the activity
|
|
if ($user->id === $initiator->id) {
|
|
continue;
|
|
}
|
|
|
|
// Prevent sending of the user does not have notification permissions
|
|
if (!$user->can('receive-notifications')) {
|
|
continue;
|
|
}
|
|
|
|
// Prevent sending if the user does not have access to the related content
|
|
$permissions = new PermissionApplicator($user);
|
|
if (!$permissions->checkOwnableUserAccess($relatedModel, 'view')) {
|
|
continue;
|
|
}
|
|
|
|
// Send the notification
|
|
try {
|
|
$user->notify(new $notification($detail, $initiator));
|
|
} catch (\Exception $exception) {
|
|
Log::error("Failed to send email notification to user [id:{$user->id}] with error: {$exception->getMessage()}");
|
|
}
|
|
}
|
|
}
|
|
}
|