mirror of
https://github.com/BookStackApp/BookStack.git
synced 2025-03-12 19:36:52 +00:00
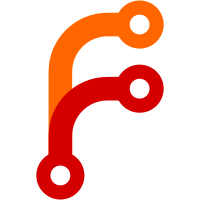
- Updated locale list - Fixed new name sorting not being case insensitive - Updated license test to account for changed deps
71 lines
2 KiB
PHP
71 lines
2 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Sorting;
|
|
|
|
use BookStack\Entities\Models\Chapter;
|
|
use BookStack\Entities\Models\Entity;
|
|
|
|
/**
|
|
* Sort comparison function for each of the possible SortSetOperation values.
|
|
* Method names should be camelCase names for the SortSetOperation enum value.
|
|
*/
|
|
class SortSetOperationComparisons
|
|
{
|
|
public static function nameAsc(Entity $a, Entity $b): int
|
|
{
|
|
return strtolower($a->name) <=> strtolower($b->name);
|
|
}
|
|
|
|
public static function nameDesc(Entity $a, Entity $b): int
|
|
{
|
|
return strtolower($b->name) <=> strtolower($a->name);
|
|
}
|
|
|
|
public static function nameNumericAsc(Entity $a, Entity $b): int
|
|
{
|
|
$numRegex = '/^\d+(\.\d+)?/';
|
|
$aMatches = [];
|
|
$bMatches = [];
|
|
preg_match($numRegex, $a->name, $aMatches);
|
|
preg_match($numRegex, $b->name, $bMatches);
|
|
$aVal = floatval(($aMatches[0] ?? 0));
|
|
$bVal = floatval(($bMatches[0] ?? 0));
|
|
|
|
return $aVal <=> $bVal;
|
|
}
|
|
|
|
public static function nameNumericDesc(Entity $a, Entity $b): int
|
|
{
|
|
return -(static::nameNumericAsc($a, $b));
|
|
}
|
|
|
|
public static function createdDateAsc(Entity $a, Entity $b): int
|
|
{
|
|
return $a->created_at->unix() <=> $b->created_at->unix();
|
|
}
|
|
|
|
public static function createdDateDesc(Entity $a, Entity $b): int
|
|
{
|
|
return $b->created_at->unix() <=> $a->created_at->unix();
|
|
}
|
|
|
|
public static function updatedDateAsc(Entity $a, Entity $b): int
|
|
{
|
|
return $a->updated_at->unix() <=> $b->updated_at->unix();
|
|
}
|
|
|
|
public static function updatedDateDesc(Entity $a, Entity $b): int
|
|
{
|
|
return $b->updated_at->unix() <=> $a->updated_at->unix();
|
|
}
|
|
|
|
public static function chaptersFirst(Entity $a, Entity $b): int
|
|
{
|
|
return ($b instanceof Chapter ? 1 : 0) - (($a instanceof Chapter) ? 1 : 0);
|
|
}
|
|
|
|
public static function chaptersLast(Entity $a, Entity $b): int
|
|
{
|
|
return ($a instanceof Chapter ? 1 : 0) - (($b instanceof Chapter) ? 1 : 0);
|
|
}
|
|
}
|