mirror of
https://github.com/crazy-max/diun.git
synced 2024-11-24 07:56:47 +00:00
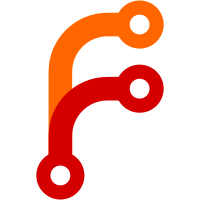
I modeled it off the Kubernetes provider a bit. It supports setting task config at group and task levels using services and meta attributes.
54 lines
802 B
Go
54 lines
802 B
Go
package nomad
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestParseServiceTags(t *testing.T) {
|
|
testCases := []struct {
|
|
input []string
|
|
expected map[string]string
|
|
}{
|
|
{
|
|
input: []string{
|
|
"noequal",
|
|
},
|
|
expected: map[string]string{},
|
|
},
|
|
{
|
|
input: []string{
|
|
"emptyequal=",
|
|
},
|
|
expected: map[string]string{
|
|
"emptyequal": "",
|
|
},
|
|
},
|
|
{
|
|
input: []string{
|
|
"key=value",
|
|
},
|
|
expected: map[string]string{
|
|
"key": "value",
|
|
},
|
|
},
|
|
{
|
|
input: []string{
|
|
"withequal=a=b",
|
|
},
|
|
expected: map[string]string{
|
|
"withequal": "a=b",
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, tt := range testCases {
|
|
tt := tt
|
|
t.Run(tt.input[0], func(t *testing.T) {
|
|
result := parseServiceTags(tt.input)
|
|
assert.Equal(t, tt.expected, result)
|
|
})
|
|
}
|
|
}
|