mirror of
https://github.com/crazy-max/diun.git
synced 2025-01-12 11:38:11 +00:00
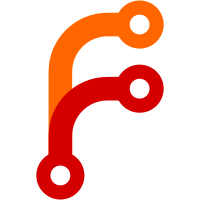
Add simple CLI to interact with Diun through gRPC Create image and notif proto services Compile and validate protos through a dedicated Dockerfile and bake target Implement proto definitions Move server as `serve` command New commands `image` and `notif` Refactor command line usage doc Better CLI error handling Tools build constraint to manage tools deps through go modules Add upgrade notes Co-authored-by: CrazyMax <crazy-max@users.noreply.github.com>
60 lines
1.4 KiB
Go
60 lines
1.4 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"runtime"
|
|
"strings"
|
|
_ "time/tzdata"
|
|
|
|
"github.com/alecthomas/kong"
|
|
"github.com/crazy-max/diun/v4/internal/model"
|
|
"github.com/rs/zerolog/log"
|
|
)
|
|
|
|
var (
|
|
version = "dev"
|
|
cli struct {
|
|
Version kong.VersionFlag
|
|
Serve ServeCmd `kong:"cmd,help='Starts Diun server.'"`
|
|
Image ImageCmd `kong:"cmd,help='Manage image manifests.'"`
|
|
Notif NotifCmd `kong:"cmd,help='Manage notifications.'"`
|
|
}
|
|
)
|
|
|
|
type Context struct {
|
|
Meta model.Meta
|
|
}
|
|
|
|
func main() {
|
|
var err error
|
|
runtime.GOMAXPROCS(runtime.NumCPU())
|
|
|
|
meta := model.Meta{
|
|
ID: "diun",
|
|
Name: "Diun",
|
|
Desc: "Docker image update notifier",
|
|
URL: "https://github.com/crazy-max/diun",
|
|
Logo: "https://raw.githubusercontent.com/crazy-max/diun/master/.res/diun.png",
|
|
Author: "CrazyMax",
|
|
Version: version,
|
|
}
|
|
meta.UserAgent = fmt.Sprintf("%s/%s go/%s %s", meta.ID, meta.Version, runtime.Version()[2:], strings.Title(runtime.GOOS))
|
|
if meta.Hostname, err = os.Hostname(); err != nil {
|
|
log.Fatal().Err(err).Msg("Cannot resolve hostname")
|
|
}
|
|
|
|
ctx := kong.Parse(&cli,
|
|
kong.Name(meta.ID),
|
|
kong.Description(fmt.Sprintf("%s. More info: %s", meta.Desc, meta.URL)),
|
|
kong.UsageOnError(),
|
|
kong.Vars{
|
|
"version": version,
|
|
},
|
|
kong.ConfigureHelp(kong.HelpOptions{
|
|
Compact: true,
|
|
Summary: true,
|
|
}))
|
|
|
|
ctx.FatalIfErrorf(ctx.Run(&Context{Meta: meta}))
|
|
}
|