mirror of
https://github.com/healthchecks/healthchecks.git
synced 2025-04-07 06:05:34 +00:00
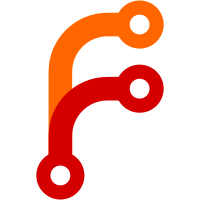
Redirect unauthenticated users to the sign in page instead. Rationale: - The content on the welcome page is what often belongs to a separate "marketing site". The marketing content is of no use on self-hosted instances, which typically have new signups disabled and are for internal use only - (the real reason, let's be honest) a number of self-hosted instances are accessible over the public internet. Search engines index the nearly identical landing pages and see them as duplicated content.
32 lines
1.2 KiB
Python
32 lines
1.2 KiB
Python
from django.test import TestCase
|
|
from django.test.utils import override_settings
|
|
|
|
|
|
class BasicsTestCase(TestCase):
|
|
@override_settings(DEBUG=False, SECRET_KEY="abc")
|
|
def test_it_redirects_to_login(self):
|
|
r = self.client.get("/")
|
|
self.assertRedirects(r, "/accounts/login/")
|
|
|
|
@override_settings(DEBUG=False, SECRET_KEY="abc")
|
|
def test_it_shows_no_warning(self):
|
|
r = self.client.get("/accounts/login/")
|
|
self.assertContains(r, "Sign In to", status_code=200)
|
|
self.assertNotContains(r, "do not use in production")
|
|
|
|
@override_settings(DEBUG=True, SECRET_KEY="abc")
|
|
def test_it_shows_debug_warning(self):
|
|
r = self.client.get("/accounts/login/")
|
|
self.assertContains(r, "Running in debug mode")
|
|
|
|
@override_settings(DEBUG=False, SECRET_KEY="---")
|
|
def test_it_shows_secret_key_warning(self):
|
|
r = self.client.get("/accounts/login/")
|
|
self.assertContains(r, "Sign In to", status_code=200)
|
|
self.assertContains(r, "Running with an insecure SECRET_KEY value")
|
|
|
|
@override_settings(REGISTRATION_OPEN=False)
|
|
def test_it_obeys_registration_open(self):
|
|
r = self.client.get("/accounts/login/")
|
|
|
|
self.assertNotContains(r, "Sign Up")
|