mirror of
https://github.com/kevinpapst/kimai2.git
synced 2025-01-10 19:47:35 +00:00
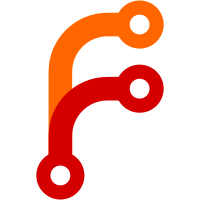
* fix column data truncated * calculate internal rate from user * show internal rate in timesheet listing * Fixed: responsivenss and size of report start page icons * fix: name display in dropdowns (and added tests) * translate reload button * fix invoice date might be in the past * fail safe customer name handling * translate invoice_date and invoice_date help * prevent URLs like start=null * prevent to reload select twice
62 lines
1.8 KiB
PHP
62 lines
1.8 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of the Kimai time-tracking app.
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace App\Tests\Form\Helper;
|
|
|
|
use App\Entity\Activity;
|
|
use App\Form\Helper\ActivityHelper;
|
|
use App\Tests\Mocks\SystemConfigurationFactory;
|
|
use PHPUnit\Framework\TestCase;
|
|
|
|
/**
|
|
* @covers \App\Form\Helper\ActivityHelper
|
|
*/
|
|
class ActivityHelperTest extends TestCase
|
|
{
|
|
private function createSut(string $format): ActivityHelper
|
|
{
|
|
$config = SystemConfigurationFactory::createStub(['activity.choice_pattern' => $format]);
|
|
$helper = new ActivityHelper($config);
|
|
|
|
return $helper;
|
|
}
|
|
|
|
public function testInvalidPattern(): void
|
|
{
|
|
$helper = $this->createSut('sdfsdf');
|
|
self::assertEquals(ActivityHelper::PATTERN_NAME, $helper->getChoicePattern());
|
|
}
|
|
|
|
public function testGetChoicePattern(): void
|
|
{
|
|
$helper = $this->createSut(
|
|
ActivityHelper::PATTERN_NAME . ActivityHelper::PATTERN_SPACER .
|
|
ActivityHelper::PATTERN_COMMENT
|
|
);
|
|
|
|
self::assertEquals(
|
|
ActivityHelper::PATTERN_NAME . ActivityHelper::SPACER .
|
|
ActivityHelper::PATTERN_COMMENT,
|
|
$helper->getChoicePattern()
|
|
);
|
|
}
|
|
|
|
public function testGetChoiceLabel(): void
|
|
{
|
|
$helper = $this->createSut(ActivityHelper::PATTERN_NAME . ActivityHelper::PATTERN_SPACER . ActivityHelper::PATTERN_COMMENT);
|
|
|
|
$activity = new Activity();
|
|
$activity->setName(' - --- - -FOO BAR- --- - - - ');
|
|
self::assertEquals('--- - -FOO BAR- ---', $helper->getChoiceLabel($activity));
|
|
|
|
$activity->setName('FOO BAR');
|
|
$activity->setComment('Lorem Ipsum');
|
|
self::assertEquals('FOO BAR - Lorem Ipsum', $helper->getChoiceLabel($activity));
|
|
}
|
|
}
|