mirror of
https://github.com/kevinpapst/kimai2.git
synced 2025-01-10 19:47:35 +00:00
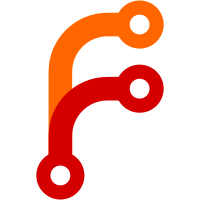
- Added: calendar entry title combination for customer, project and activity - Added: show not_invoiced and not_exported data in detail screens - Added: force logout if user is disabled - Added: reduced amount of database queries on several screens - Fixed: open-close status on work-contract screen for users without configuration - Fixed: failsafe order/orderBy in query if manually manipulated to be null - Fixed: unify statistic calculation (not_invoiced and not_exported) across screens - Tech: bump packages - Tech: Symfony 6.4
85 lines
2.9 KiB
PHP
85 lines
2.9 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of the Kimai time-tracking app.
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace App\Tests\Reporting\ProjectView;
|
|
|
|
use App\Entity\Project;
|
|
use App\Model\ProjectBudgetStatisticModel;
|
|
use App\Model\Statistic\BudgetStatistic;
|
|
use App\Reporting\ProjectView\ProjectViewModel;
|
|
use PHPUnit\Framework\TestCase;
|
|
|
|
/**
|
|
* @covers \App\Reporting\ProjectView\ProjectViewModel
|
|
*/
|
|
class ProjectViewModelTest extends TestCase
|
|
{
|
|
public function testDefaults(): void
|
|
{
|
|
$project = new Project();
|
|
$model = new ProjectBudgetStatisticModel($project);
|
|
$total = new BudgetStatistic();
|
|
$model->setStatisticTotal($total);
|
|
$sut = new ProjectViewModel($model);
|
|
|
|
self::assertSame($project, $sut->getProject());
|
|
self::assertSame(0, $sut->getDurationDay());
|
|
self::assertSame(0, $sut->getDurationMonth());
|
|
self::assertSame(0, $sut->getDurationTotal());
|
|
self::assertSame(0, $sut->getDurationWeek());
|
|
self::assertSame(0, $sut->getNotExportedDuration());
|
|
self::assertSame(0.0, $sut->getNotExportedRate());
|
|
self::assertSame(0.0, $sut->getRateTotal());
|
|
self::assertNull($sut->getLastRecord());
|
|
self::assertNull($sut->getLastRecord());
|
|
self::assertSame(0.0, $sut->getBillableRate());
|
|
self::assertSame(0, $sut->getBillableDuration());
|
|
}
|
|
|
|
public function testSetterGetter(): void
|
|
{
|
|
$project = new Project();
|
|
|
|
$model = new ProjectBudgetStatisticModel($project);
|
|
$total = new BudgetStatistic();
|
|
$total->setCounter(123);
|
|
$total->setDuration(3456789);
|
|
$total->setDurationBillable(3456789);
|
|
$total->setDurationBillableExported(3400000);
|
|
$total->setRate(789.0);
|
|
$total->setRateBillable(16789.0);
|
|
$total->setRateBillableExported(10000.0);
|
|
$model->setStatisticTotal($total);
|
|
|
|
$sut = new ProjectViewModel($model);
|
|
|
|
$date = new \DateTime();
|
|
|
|
$sut->setDurationDay(123456789);
|
|
$sut->setDurationMonth(23456789);
|
|
$sut->setDurationWeek(456789);
|
|
$sut->setLastRecord($date);
|
|
|
|
self::assertSame(123456789, $sut->getDurationDay());
|
|
self::assertSame(23456789, $sut->getDurationMonth());
|
|
self::assertSame(3456789, $sut->getDurationTotal());
|
|
self::assertSame(456789, $sut->getDurationWeek());
|
|
self::assertSame(56789, $sut->getNotExportedDuration());
|
|
self::assertSame(6789.0, $sut->getNotExportedRate());
|
|
self::assertSame(789.0, $sut->getRateTotal());
|
|
self::assertSame($date, $sut->getLastRecord());
|
|
|
|
$date = new \DateTime();
|
|
$sut->setLastRecord($date);
|
|
|
|
self::assertSame($date, $sut->getLastRecord());
|
|
self::assertSame(16789.0, $sut->getBillableRate());
|
|
self::assertSame(3456789, $sut->getBillableDuration());
|
|
}
|
|
}
|