mirror of
https://github.com/kevinpapst/kimai2.git
synced 2024-12-22 12:18:29 +00:00
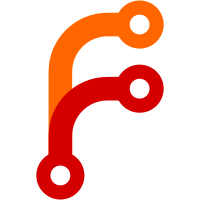
* bump version * fix translation ids * added css classes to modify form with custom css * improve export pdf file names * respect financial year in new report * added new InvoiceCalculator: price * upgrade packages and node-sass to v7 * title pattern for customer, project and activity via API * support negative money without currency * fix sub-locale in print export template * fix overbooking validation for monthly budget * fix copying entities with different set of custom-fields compared to the current configuration
46 lines
1.3 KiB
PHP
46 lines
1.3 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of the Kimai time-tracking app.
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace App\Tests\Mocks;
|
|
|
|
use App\Entity\ProjectMeta;
|
|
use App\Event\ProjectMetaDefinitionEvent;
|
|
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
|
|
use Symfony\Component\Form\Extension\Core\Type\IntegerType;
|
|
use Symfony\Component\Form\Extension\Core\Type\TextType;
|
|
use Symfony\Component\Validator\Constraints\Length;
|
|
|
|
class ProjectTestMetaFieldSubscriberMock implements EventSubscriberInterface
|
|
{
|
|
public static function getSubscribedEvents(): array
|
|
{
|
|
return [
|
|
ProjectMetaDefinitionEvent::class => ['loadMeta', 200],
|
|
];
|
|
}
|
|
|
|
public function loadMeta(ProjectMetaDefinitionEvent $event)
|
|
{
|
|
$definition = (new ProjectMeta())
|
|
->setName('metatestmock')
|
|
->setType(TextType::class)
|
|
->addConstraint(new Length(['max' => 200]))
|
|
->setIsVisible(true);
|
|
|
|
$event->getEntity()->setMetaField($definition);
|
|
|
|
$definition = (new ProjectMeta())
|
|
->setName('foobar')
|
|
->setType(IntegerType::class)
|
|
->setIsVisible(false);
|
|
|
|
$event->getEntity()->setMetaField($definition);
|
|
}
|
|
}
|