mirror of
https://github.com/netdata/netdata.git
synced 2025-04-13 01:08:11 +00:00
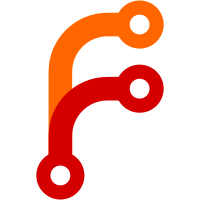
* listening ipv6 sockets may be both ipv4 and ipv6, depending on the IPV6_ONLY flag * working libmnl ipv46 detection and added latency and retransmits from TCP_INFO * fix aggregations for rtt and retrans * code cleanup * code cleanup * code cleanup again * restore field renames * count namespaces * run namespaces in parallel * add libmnl to buildinfo * lock around safe_fork() * libmnl ports are in network byte order * posix spawn server for both executables and callback functions * local-sockets and network-viewer using the new spawn server * cleanup spawn servers sockets * spawn server stdin and stdout are linked to /dev/null * no need for spinlock in spawn server * empty all parameters * new spawn server is now used for plugins.d plugins * fix for environ * claiming script runs via the new spawn server * tc.plugin uses the new spawn server * analytics, buildinfo and cgroups.plugin use the new spawn server * cgroup-discovery uses the new spawn server * added ability to wait or kill spawned processes * removed old spawn server and now alert notifications use the new one * remove left-overs * hide spawn server internals; started working on windows version of the spawn server * fixes for windows * more windows work * more work on windows * added debug log to spawn server * fix compilation warnings * enable static threads on windows * running external plugins * working spawn server on windows * spawn server logs to collectoers.log * log windows last error together with errno * log updates * cleanup * decode_argv does not add an empty parameter * removed debug log * removed debug return * rework on close_range() * eliminate the need for waitid() * clear errno on the signal handler * added universal os_setproctitle() call to support FreeBSD too * os_get_pid_max() for windows and macos * isolate pids array from the rest of the code in apps.plugin so that it can be turned to a hashtable
211 lines
5.4 KiB
C
211 lines
5.4 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#include "common.h"
|
|
|
|
void *aclk_main(void *ptr);
|
|
void *analytics_main(void *ptr);
|
|
void *cpuidlejitter_main(void *ptr);
|
|
void *global_statistics_main(void *ptr);
|
|
void *global_statistics_extended_main(void *ptr);
|
|
void *health_main(void *ptr);
|
|
void *pluginsd_main(void *ptr);
|
|
void *service_main(void *ptr);
|
|
void *statsd_main(void *ptr);
|
|
void *profile_main(void *ptr);
|
|
void *replication_thread_main(void *ptr);
|
|
|
|
extern bool global_statistics_enabled;
|
|
|
|
const struct netdata_static_thread static_threads_common[] = {
|
|
{
|
|
.name = "P[idlejitter]",
|
|
.config_section = CONFIG_SECTION_PLUGINS,
|
|
.config_name = "idlejitter",
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = cpuidlejitter_main
|
|
},
|
|
{
|
|
.name = "HEALTH",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = health_main
|
|
},
|
|
{
|
|
.name = "ANALYTICS",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = analytics_main
|
|
},
|
|
{
|
|
.name = "STATS_GLOBAL",
|
|
.config_section = CONFIG_SECTION_PLUGINS,
|
|
.config_name = "netdata monitoring",
|
|
.env_name = "NETDATA_INTERNALS_MONITORING",
|
|
.global_variable = &global_statistics_enabled,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = global_statistics_main
|
|
},
|
|
{
|
|
.name = "STATS_GLOBAL_EXT",
|
|
.config_section = CONFIG_SECTION_PLUGINS,
|
|
.config_name = "netdata monitoring extended",
|
|
.env_name = "NETDATA_INTERNALS_EXTENDED_MONITORING",
|
|
.global_variable = &global_statistics_enabled,
|
|
.enabled = 0, // this is ignored - check main() for "netdata monitoring extended"
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = global_statistics_extended_main
|
|
},
|
|
{
|
|
.name = "PLUGINSD",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = pluginsd_main
|
|
},
|
|
{
|
|
.name = "SERVICE",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = service_main
|
|
},
|
|
{
|
|
.name = "STATSD_FLUSH",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = statsd_main
|
|
},
|
|
{
|
|
.name = "EXPORTING",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = exporting_main
|
|
},
|
|
{
|
|
.name = "SNDR[localhost]",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = rrdpush_sender_thread
|
|
},
|
|
{
|
|
.name = "WEB[1]",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = socket_listen_main_static_threaded
|
|
},
|
|
|
|
#ifdef ENABLE_H2O
|
|
{
|
|
.name = "h2o",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = h2o_main
|
|
},
|
|
#endif
|
|
|
|
#ifdef ENABLE_ACLK
|
|
{
|
|
.name = "ACLK_MAIN",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = aclk_main
|
|
},
|
|
#endif
|
|
|
|
{
|
|
.name = "RRDCONTEXT",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = rrdcontext_main
|
|
},
|
|
|
|
{
|
|
.name = "REPLAY[1]",
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.enabled = 1,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = replication_thread_main
|
|
},
|
|
{
|
|
.name = "P[PROFILE]",
|
|
.config_section = CONFIG_SECTION_PLUGINS,
|
|
.config_name = "profile",
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = profile_main
|
|
},
|
|
|
|
// terminator
|
|
{
|
|
.name = NULL,
|
|
.config_section = NULL,
|
|
.config_name = NULL,
|
|
.env_name = NULL,
|
|
.enabled = 0,
|
|
.thread = NULL,
|
|
.init_routine = NULL,
|
|
.start_routine = NULL
|
|
}
|
|
};
|
|
|
|
struct netdata_static_thread *
|
|
static_threads_concat(const struct netdata_static_thread *lhs,
|
|
const struct netdata_static_thread *rhs)
|
|
{
|
|
struct netdata_static_thread *res;
|
|
|
|
int lhs_size = 0;
|
|
for (; lhs[lhs_size].name; lhs_size++) {}
|
|
|
|
int rhs_size = 0;
|
|
for (; rhs[rhs_size].name; rhs_size++) {}
|
|
|
|
res = callocz(lhs_size + rhs_size + 1, sizeof(struct netdata_static_thread));
|
|
|
|
for (int i = 0; i != lhs_size; i++)
|
|
memcpy(&res[i], &lhs[i], sizeof(struct netdata_static_thread));
|
|
|
|
for (int i = 0; i != rhs_size; i++)
|
|
memcpy(&res[lhs_size + i], &rhs[i], sizeof(struct netdata_static_thread));
|
|
|
|
return res;
|
|
}
|