mirror of
https://github.com/netdata/netdata.git
synced 2025-05-01 07:59:52 +00:00
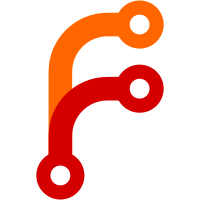
* modularized all external plugins * added README.md in plugins * fixed title * fixed typo * relative link to external plugins * external plugins configuration README * added plugins link * remove plugins link * plugin names are links * added links to external plugins * removed unecessary spacing * list to table * added language * fixed typo * list to table on internal plugins * added more documentation to internal plugins * moved python, node, and bash code and configs into the external plugins * added statsd README * fix bug with corrupting config.h every 2nd compilation * moved all config files together with their code * more documentation * diskspace info * fixed broken links in apps.plugin * added backends docs * updated plugins readme * move nc-backend.sh to backends * created daemon directory * moved all code outside src/ * fixed readme identation * renamed plugins.d.plugin to plugins.d * updated readme * removed linux- from linux plugins * updated readme * updated readme * updated readme * updated readme * updated readme * updated readme * fixed README.md links * fixed netdata tree links * updated codacy, codeclimate and lgtm excluded paths * update CMakeLists.txt * updated automake options at top directory * libnetdata slit into directories * updated READMEs * updated READMEs * updated ARL docs * updated ARL docs * moved /plugins to /collectors * moved all external plugins outside plugins.d * updated codacy, codeclimate, lgtm * updated README * updated url * updated readme * updated readme * updated readme * updated readme * moved api and web into webserver * web/api web/gui web/server * modularized webserver * removed web/gui/version.txt
88 lines
2.6 KiB
C
88 lines
2.6 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#ifndef NETDATA_EVAL_H
|
|
#define NETDATA_EVAL_H 1
|
|
|
|
#include "../libnetdata.h"
|
|
|
|
#define EVAL_MAX_VARIABLE_NAME_LENGTH 300
|
|
|
|
typedef enum rrdcalc_status {
|
|
RRDCALC_STATUS_REMOVED = -2,
|
|
RRDCALC_STATUS_UNDEFINED = -1,
|
|
RRDCALC_STATUS_UNINITIALIZED = 0,
|
|
RRDCALC_STATUS_CLEAR = 1,
|
|
RRDCALC_STATUS_RAISED = 2,
|
|
RRDCALC_STATUS_WARNING = 3,
|
|
RRDCALC_STATUS_CRITICAL = 4
|
|
} RRDCALC_STATUS;
|
|
|
|
typedef struct eval_variable {
|
|
char *name;
|
|
uint32_t hash;
|
|
struct eval_variable *next;
|
|
} EVAL_VARIABLE;
|
|
|
|
typedef struct eval_expression {
|
|
const char *source;
|
|
const char *parsed_as;
|
|
|
|
RRDCALC_STATUS *status;
|
|
calculated_number *this;
|
|
time_t *after;
|
|
time_t *before;
|
|
|
|
calculated_number result;
|
|
|
|
int error;
|
|
BUFFER *error_msg;
|
|
|
|
// hidden EVAL_NODE *
|
|
void *nodes;
|
|
|
|
// custom data to be used for looking up variables
|
|
struct rrdcalc *rrdcalc;
|
|
} EVAL_EXPRESSION;
|
|
|
|
#define EVAL_VALUE_INVALID 0
|
|
#define EVAL_VALUE_NUMBER 1
|
|
#define EVAL_VALUE_VARIABLE 2
|
|
#define EVAL_VALUE_EXPRESSION 3
|
|
|
|
// parsing and evaluation
|
|
#define EVAL_ERROR_OK 0
|
|
|
|
// parsing errors
|
|
#define EVAL_ERROR_MISSING_CLOSE_SUBEXPRESSION 1
|
|
#define EVAL_ERROR_UNKNOWN_OPERAND 2
|
|
#define EVAL_ERROR_MISSING_OPERAND 3
|
|
#define EVAL_ERROR_MISSING_OPERATOR 4
|
|
#define EVAL_ERROR_REMAINING_GARBAGE 5
|
|
#define EVAL_ERROR_IF_THEN_ELSE_MISSING_ELSE 6
|
|
|
|
// evaluation errors
|
|
#define EVAL_ERROR_INVALID_VALUE 101
|
|
#define EVAL_ERROR_INVALID_NUMBER_OF_OPERANDS 102
|
|
#define EVAL_ERROR_VALUE_IS_NAN 103
|
|
#define EVAL_ERROR_VALUE_IS_INFINITE 104
|
|
#define EVAL_ERROR_UNKNOWN_VARIABLE 105
|
|
|
|
// parse the given string as an expression and return:
|
|
// a pointer to an expression if it parsed OK
|
|
// NULL in which case the pointer to error has the error code
|
|
extern EVAL_EXPRESSION *expression_parse(const char *string, const char **failed_at, int *error);
|
|
|
|
// free all resources allocated for an expression
|
|
extern void expression_free(EVAL_EXPRESSION *expression);
|
|
|
|
// convert an error code to a message
|
|
extern const char *expression_strerror(int error);
|
|
|
|
// evaluate an expression and return
|
|
// 1 = OK, the result is in: expression->result
|
|
// 2 = FAILED, the error message is in: buffer_tostring(expression->error_msg)
|
|
extern int expression_evaluate(EVAL_EXPRESSION *expression);
|
|
|
|
extern int health_variable_lookup(const char *variable, uint32_t hash, struct rrdcalc *rc, calculated_number *result);
|
|
|
|
#endif //NETDATA_EVAL_H
|