mirror of
https://github.com/netdata/netdata.git
synced 2025-05-15 22:10:42 +00:00
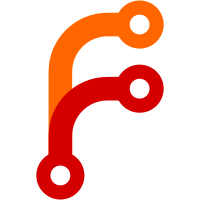
* squashed and rebased to master * fix overflow and single character bug in sanitize; include rrd.h instead of node_info.h * added unittest for UTF-8 multibyte sanitization * Fix unit test compilation * Fix CMake build * remove double sanitizer for opentsdb; cleanup sanitize_json_string() * rename error_description to error_message to avoid conflict with json-c * revert last and undef error_description from json-c * more unittests; attempt to fix protobuf map issue * get rid of rrdlabels_get() and replace it with a safe version that writes the value to a buffer * added dictionary sorting unittest; rrdlabels_to_buffer() now is sorted * better sorted dictionary checking * proper unittesting for sorted dictionaries * call dictionary deletion callback when destroying the dictionary * remove obsolete variable * Fix exporting unit tests * Fix k8s label parsing test * workaround for cmocka and strdupz() * Bypass cmocka memory allocation check * Revert "Bypass cmocka memory allocation check" This reverts commit4c49923839
. * Revert "workaround for cmocka and strdupz()" This reverts commit7bebee0480
. * Bypass cmocka memory allocation checks * respect json formatting for chart labels * cloud sends colons * print the value only once * allow parenthesis in values and spaces; make stream sender send quotes for values Co-authored-by: Vladimir Kobal <vlad@prokk.net>
85 lines
2.3 KiB
C
85 lines
2.3 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#include "exporting_engine.h"
|
|
|
|
/**
|
|
* Clean the instance config.
|
|
*
|
|
* @param config an instance config structure.
|
|
*/
|
|
static void clean_instance_config(struct instance_config *config)
|
|
{
|
|
if(!config)
|
|
return;
|
|
|
|
freez((void *)config->type_name);
|
|
freez((void *)config->name);
|
|
freez((void *)config->destination);
|
|
freez((void *)config->username);
|
|
freez((void *)config->password);
|
|
freez((void *)config->prefix);
|
|
freez((void *)config->hostname);
|
|
|
|
simple_pattern_free(config->charts_pattern);
|
|
|
|
simple_pattern_free(config->hosts_pattern);
|
|
}
|
|
|
|
/**
|
|
* Clean the allocated variables
|
|
*
|
|
* @param instance an instance data structure.
|
|
*/
|
|
void clean_instance(struct instance *instance)
|
|
{
|
|
clean_instance_config(&instance->config);
|
|
buffer_free(instance->labels_buffer);
|
|
|
|
uv_cond_destroy(&instance->cond_var);
|
|
// uv_mutex_destroy(&instance->mutex);
|
|
}
|
|
|
|
/**
|
|
* Clean up a simple connector instance on Netdata exit
|
|
*
|
|
* @param instance an instance data structure.
|
|
*/
|
|
void simple_connector_cleanup(struct instance *instance)
|
|
{
|
|
info("EXPORTING: cleaning up instance %s ...", instance->config.name);
|
|
|
|
struct simple_connector_data *simple_connector_data =
|
|
(struct simple_connector_data *)instance->connector_specific_data;
|
|
|
|
freez(simple_connector_data->auth_string);
|
|
|
|
buffer_free(instance->buffer);
|
|
buffer_free(simple_connector_data->buffer);
|
|
buffer_free(simple_connector_data->header);
|
|
|
|
struct simple_connector_buffer *next_buffer = simple_connector_data->first_buffer;
|
|
for (int i = 0; i < instance->config.buffer_on_failures; i++) {
|
|
struct simple_connector_buffer *current_buffer = next_buffer;
|
|
next_buffer = next_buffer->next;
|
|
|
|
buffer_free(current_buffer->header);
|
|
buffer_free(current_buffer->buffer);
|
|
freez(current_buffer);
|
|
}
|
|
|
|
#ifdef ENABLE_HTTPS
|
|
if (simple_connector_data->conn)
|
|
SSL_free(simple_connector_data->conn);
|
|
#endif
|
|
|
|
freez(simple_connector_data);
|
|
|
|
struct simple_connector_config *simple_connector_config =
|
|
(struct simple_connector_config *)instance->config.connector_specific_config;
|
|
freez(simple_connector_config);
|
|
|
|
info("EXPORTING: instance %s exited", instance->config.name);
|
|
instance->exited = 1;
|
|
|
|
return;
|
|
}
|