mirror of
https://github.com/netdata/netdata.git
synced 2025-05-04 01:10:03 +00:00
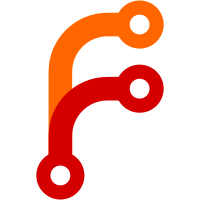
* Remove code for bundling the dashoard on install. * Bundle the dashboard code directly into the agent repo. This diffstat looks huge, but it’s actually relatively simple. The only _actual_ changes are in the Makefiles, `configure.ac`, and the addition of `generate_dashboard_makefile.py`. Everything else consists of removing files that are included in the dashboard tarball, and extracting the contents of the tarball into `web/gui/dashboard`. * CI cleanup. * Automate bundling of the dashboard code. This replaces the makefile generator script with one that handles bundling of the dashboard code in it’s entirety, and updates the GHA workflow used for generating dashboard PRs to use that instead of the existing shell commands. It also removes the packaging/dashboard.* files, as they are no longer needed.
105 lines
2.6 KiB
Python
Executable file
105 lines
2.6 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
#
|
|
# Copyright: © 2021 Netdata Inc.
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
'''Bundle the dashboard code into the agent repo.'''
|
|
|
|
import os
|
|
import shutil
|
|
import subprocess
|
|
import sys
|
|
|
|
from pathlib import Path
|
|
|
|
os.chdir(Path(__file__).parent.absolute())
|
|
|
|
BASEPATH = Path('dashboard')
|
|
|
|
URLTEMPLATE = 'https://github.com/netdata/dashboard/releases/download/{0}/dashboard.tar.gz'
|
|
|
|
MAKEFILETEMPLATE = '''
|
|
# Auto-generated by generate-dashboard-makefile.py
|
|
# Copyright: © 2021 Netdata Inc.
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
MAINTAINERCLEANFILES = $(srcdir)/Makefile.in
|
|
|
|
dist_noinst_DATA = \\
|
|
README.md
|
|
|
|
dist_web_DATA = \\
|
|
{0} \\
|
|
$(NULL)
|
|
|
|
webcssdir=$(webdir)/css
|
|
dist_webcss_DATA = \\
|
|
{1} \\
|
|
$(NULL)
|
|
|
|
webfontsdir=$(webdir)/fonts
|
|
dist_webfonts_DATA = \\
|
|
{2} \\
|
|
$(NULL)
|
|
|
|
webimagesdir=$(webdir)/images
|
|
dist_webimages_DATA = \\
|
|
{3} \\
|
|
$(NULL)
|
|
|
|
weblibdir=$(webdir)/lib
|
|
dist_weblib_DATA = \\
|
|
{4} \\
|
|
$(NULL)
|
|
|
|
webstaticcssdir=$(webdir)/static/css
|
|
dist_webstaticcss_DATA = \\
|
|
{5} \\
|
|
$(NULL)
|
|
|
|
webstaticjsdir=$(webdir)/static/js
|
|
dist_webstaticjs_DATA = \\
|
|
{6} \\
|
|
$(NULL)
|
|
|
|
webstaticmediadir=$(webdir)/static/media
|
|
dist_webstaticmedia_DATA = \\
|
|
{7} \\
|
|
$(NULL)
|
|
'''
|
|
|
|
|
|
def copy_dashboard(tag):
|
|
'''Fetch and bundle the dashboard code.'''
|
|
shutil.rmtree(BASEPATH)
|
|
BASEPATH.mkdir()
|
|
subprocess.check_call('curl -L -o dashboard.tar.gz ' + URLTEMPLATE.format(tag), shell=True)
|
|
subprocess.check_call('tar -xvzf dashboard.tar.gz -C ' + str(BASEPATH) + ' --strip-components=1', shell=True)
|
|
BASEPATH.joinpath('README.md').symlink_to('../.dashboard-notice.md')
|
|
# BASEPATH.joinpath('..', 'dashboard.tar.gz').unlink()
|
|
|
|
|
|
def genfilelist(path):
|
|
'''Generate a list of files for the Makefile.'''
|
|
files = [f for f in path.iterdir() if f.is_file() and f.name != 'README.md']
|
|
files = [Path(*f.parts[1:]) for f in files]
|
|
files.sort()
|
|
return ' \\\n '.join([str(f) for f in files])
|
|
|
|
|
|
def write_makefile():
|
|
'''Write out the makefile for the dashboard code.'''
|
|
MAKEFILEDATA = MAKEFILETEMPLATE.format(
|
|
genfilelist(BASEPATH),
|
|
genfilelist(BASEPATH.joinpath('css')),
|
|
genfilelist(BASEPATH.joinpath('fonts')),
|
|
genfilelist(BASEPATH.joinpath('images')),
|
|
genfilelist(BASEPATH.joinpath('lib')),
|
|
genfilelist(BASEPATH.joinpath('static', 'css')),
|
|
genfilelist(BASEPATH.joinpath('static', 'js')),
|
|
genfilelist(BASEPATH.joinpath('static', 'media')),
|
|
)
|
|
|
|
BASEPATH.joinpath('Makefile.am').write_text(MAKEFILEDATA)
|
|
|
|
|
|
copy_dashboard(sys.argv[1])
|
|
write_makefile()
|