mirror of
https://github.com/netdata/netdata.git
synced 2025-04-26 22:04:46 +00:00
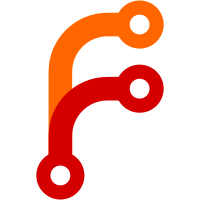
* initial webrtc setup * missing files * rewrite of webrtc integration * initialization and cleanup of webrtc connections * make it compile without libdatachannel * add missing webrtc_initialize() function when webrtc is not enabled * make c++17 optional * add build/m4/ax_compiler_vendor.m4 * add ax_cxx_compile_stdcxx.m4 * added new m4 files to makefile.am * id all webrtc connections * show warning when webrtc is disabled * fixed message * moved all webrtc error checking inside webrtc.cpp * working webrtc connection establishment and cleanup * remove obsolete code * rewrote webrtc code in C to remove dependency for c++17 * fixed left-over reference * detect binary and text messages * minor fix * naming of webrtc threads * added webrtc configuration * fix for thread_get_name_np() * smaller web_client memory footprint * universal web clients cache * free web clients every 100 uses * webrtc is now enabled by default only when compiled with internal checks * webrtc responses to /api/ requests, including LZ4 compression * fix for binary and text messages * web_client_cache is now global * unification of the internal web server API, for web requests, aclk request, webrtc requests * more cleanup and unification of web client timings * fixed compiler warnings * update sent and received bytes * eliminated of almost all big buffers in web client * registry now uses the new json generation * cookies are now an array; fixed redirects * fix redirects, again * write cookies directly to the header buffer, eliminating the need for cookie structures in web client * reset the has_cookies flag * gathered all web client cleanup to one function * fixes redirects * added summary.globals in /api/v2/data response * ars to arc in /api/v2/data * properly handle host impersonation * set the context of mem.numa_nodes
96 lines
2.9 KiB
C
96 lines
2.9 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#ifndef NETDATA_SQLITE_ACLK_H
|
|
#define NETDATA_SQLITE_ACLK_H
|
|
|
|
#include "sqlite3.h"
|
|
|
|
|
|
#ifndef ACLK_MAX_CHART_BATCH
|
|
#define ACLK_MAX_CHART_BATCH (200)
|
|
#endif
|
|
#ifndef ACLK_MAX_CHART_BATCH_COUNT
|
|
#define ACLK_MAX_CHART_BATCH_COUNT (10)
|
|
#endif
|
|
#define ACLK_MAX_ALERT_UPDATES (5)
|
|
#define ACLK_DATABASE_CLEANUP_FIRST (1200)
|
|
#define ACLK_DATABASE_CLEANUP_INTERVAL (3600)
|
|
#define ACLK_DELETE_ACK_ALERTS_INTERNAL (86400)
|
|
#define ACLK_SYNC_QUERY_SIZE 512
|
|
|
|
static inline void uuid_unparse_lower_fix(uuid_t *uuid, char *out)
|
|
{
|
|
uuid_unparse_lower(*uuid, out);
|
|
out[8] = '_';
|
|
out[13] = '_';
|
|
out[18] = '_';
|
|
out[23] = '_';
|
|
}
|
|
|
|
static inline int claimed()
|
|
{
|
|
return localhost->aclk_state.claimed_id != NULL;
|
|
}
|
|
|
|
|
|
#define TABLE_ACLK_ALERT "CREATE TABLE IF NOT EXISTS aclk_alert_%s (sequence_id INTEGER PRIMARY KEY, " \
|
|
"alert_unique_id, date_created, date_submitted, date_cloud_ack, filtered_alert_unique_id NOT NULL, " \
|
|
"unique(alert_unique_id));"
|
|
|
|
#define INDEX_ACLK_ALERT "CREATE INDEX IF NOT EXISTS aclk_alert_index_%s ON aclk_alert_%s (alert_unique_id);"
|
|
enum aclk_database_opcode {
|
|
ACLK_DATABASE_NOOP = 0,
|
|
|
|
ACLK_DATABASE_ALARM_HEALTH_LOG,
|
|
ACLK_DATABASE_CLEANUP,
|
|
ACLK_DATABASE_DELETE_HOST,
|
|
ACLK_DATABASE_NODE_STATE,
|
|
ACLK_DATABASE_PUSH_ALERT,
|
|
ACLK_DATABASE_PUSH_ALERT_CONFIG,
|
|
ACLK_DATABASE_PUSH_ALERT_SNAPSHOT,
|
|
ACLK_DATABASE_QUEUE_REMOVED_ALERTS,
|
|
ACLK_DATABASE_TIMER,
|
|
|
|
// leave this last
|
|
// we need it to check for worker utilization
|
|
ACLK_MAX_ENUMERATIONS_DEFINED
|
|
};
|
|
|
|
struct aclk_database_cmd {
|
|
enum aclk_database_opcode opcode;
|
|
void *param[2];
|
|
struct completion *completion;
|
|
};
|
|
|
|
#define ACLK_DATABASE_CMD_Q_MAX_SIZE (1024)
|
|
|
|
struct aclk_database_cmdqueue {
|
|
unsigned head, tail;
|
|
struct aclk_database_cmd cmd_array[ACLK_DATABASE_CMD_Q_MAX_SIZE];
|
|
};
|
|
|
|
struct aclk_sync_host_config {
|
|
RRDHOST *host;
|
|
int alert_updates;
|
|
time_t node_info_send_time;
|
|
time_t node_collectors_send;
|
|
char uuid_str[UUID_STR_LEN];
|
|
char node_id[UUID_STR_LEN];
|
|
uint64_t alerts_batch_id; // batch id for alerts to use
|
|
uint64_t alerts_start_seq_id; // cloud has asked to start streaming from
|
|
uint64_t alerts_snapshot_id; // will contain the snapshot_id value if snapshot was requested
|
|
uint64_t alerts_ack_sequence_id; // last sequence_id ack'ed from cloud via sendsnapshot message
|
|
};
|
|
|
|
extern sqlite3 *db_meta;
|
|
|
|
int aclk_database_enq_cmd_noblock(struct aclk_database_cmd *cmd);
|
|
void sql_create_aclk_table(RRDHOST *host, uuid_t *host_uuid, uuid_t *node_id);
|
|
void sql_aclk_sync_init(void);
|
|
void aclk_push_alert_config(const char *node_id, const char *config_hash);
|
|
void aclk_push_node_alert_snapshot(const char *node_id);
|
|
void aclk_push_node_health_log(const char *node_id);
|
|
void aclk_push_node_removed_alerts(const char *node_id);
|
|
void schedule_node_info_update(RRDHOST *host);
|
|
|
|
#endif //NETDATA_SQLITE_ACLK_H
|