mirror of
https://github.com/netdata/netdata.git
synced 2025-04-25 21:43:55 +00:00
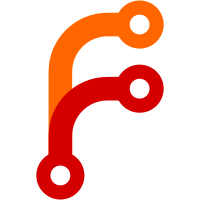
* apache units fix * beanstalk * bind_rndc * boinc * ceph * chrony * couchdb * dns_query * dnsdist * dockerd * dovecot * elasticsearch by @vlvkobal <3 * example * exim * fail2ban * freeradius minor fixes * freeradius minor fixes * freeradius minor fixes * go_expvar * haproxy * hddtemp * httpcheck * icecast * ipfs * isc_dhcpd * litespeed * logind * megacli * memcached * mongodb * monit * mysql * nginx * nginx_plus * nsd * ntpd * nvidia_smi * openldap * ovpn_status * phpfm * portcheck * postfix * postgres * powerdns * proxysql * puppet * rabbitmq * redis * restroshare * samba * sensors * smartdlog * spigotmc * springboot * squid * retroshare * tomcat * retroshare * tor * traefik * traefik * unbound * uwsgi * varnish * w1sensor * web_log * ok codacy * retroshare * ipfs
79 lines
2.1 KiB
Python
79 lines
2.1 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Description: RetroShare netdata python.d module
|
|
# Authors: sehraf
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
import json
|
|
|
|
from bases.FrameworkServices.UrlService import UrlService
|
|
|
|
|
|
ORDER = [
|
|
'bandwidth',
|
|
'peers',
|
|
'dht',
|
|
]
|
|
|
|
CHARTS = {
|
|
'bandwidth': {
|
|
'options': [None, 'RetroShare Bandwidth', 'kilobits/s', 'RetroShare', 'retroshare.bandwidth', 'area'],
|
|
'lines': [
|
|
['bandwidth_up_kb', 'Upload'],
|
|
['bandwidth_down_kb', 'Download']
|
|
]
|
|
},
|
|
'peers': {
|
|
'options': [None, 'RetroShare Peers', 'peers', 'RetroShare', 'retroshare.peers', 'line'],
|
|
'lines': [
|
|
['peers_all', 'All friends'],
|
|
['peers_connected', 'Connected friends']
|
|
]
|
|
},
|
|
'dht': {
|
|
'options': [None, 'Retroshare DHT', 'peers', 'RetroShare', 'retroshare.dht', 'line'],
|
|
'lines': [
|
|
['dht_size_all', 'DHT nodes estimated'],
|
|
['dht_size_rs', 'RS nodes estimated']
|
|
]
|
|
}
|
|
}
|
|
|
|
|
|
class Service(UrlService):
|
|
def __init__(self, configuration=None, name=None):
|
|
UrlService.__init__(self, configuration=configuration, name=name)
|
|
self.order = ORDER
|
|
self.definitions = CHARTS
|
|
self.baseurl = self.configuration.get('url', 'http://localhost:9090')
|
|
|
|
def _get_stats(self):
|
|
"""
|
|
Format data received from http request
|
|
:return: dict
|
|
"""
|
|
try:
|
|
raw = self._get_raw_data()
|
|
parsed = json.loads(raw)
|
|
if str(parsed['returncode']) != 'ok':
|
|
return None
|
|
except (TypeError, ValueError):
|
|
return None
|
|
|
|
return parsed['data'][0]
|
|
|
|
def _get_data(self):
|
|
"""
|
|
Get data from API
|
|
:return: dict
|
|
"""
|
|
self.url = self.baseurl + '/api/v2/stats'
|
|
data = self._get_stats()
|
|
if data is None:
|
|
return None
|
|
|
|
data['bandwidth_up_kb'] = data['bandwidth_up_kb'] * -1
|
|
if data['dht_active'] is False:
|
|
data['dht_size_all'] = None
|
|
data['dht_size_rs'] = None
|
|
|
|
return data
|