mirror of
https://github.com/netdata/netdata.git
synced 2025-04-06 06:25:32 +00:00
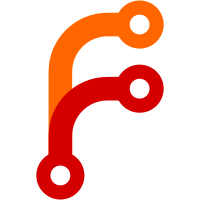
* rrdset - in progress * rrdset optimal constructor; rrdset conflict * rrdset final touches * re-organization of rrdset object members * prevent use-after-free * dictionary dfe supports also counting of iterations * rrddim managed by dictionary * rrd.h cleanup * DICTIONARY_ITEM now is referencing actual dictionary items in the code * removed rrdset linked list * Revert "removed rrdset linked list" This reverts commit 690d6a588b4b99619c2c5e10f84e8f868ae6def5. * removed rrdset linked list * added comments * Switch chart uuid to static allocation in rrdset Remove unused functions * rrdset_archive() and friends... * always create rrdfamily * enable ml_free_dimension * rrddim_foreach done with dfe * most custom rrddim loops replaced with rrddim_foreach * removed accesses to rrddim->dimensions * removed locks that are no longer needed * rrdsetvar is now managed by the dictionary * set rrdset is rrdsetvar, fixes https://github.com/netdata/netdata/pull/13646#issuecomment-1242574853 * conflict callback of rrdsetvar now properly checks if it has to reset the variable * dictionary registered callbacks accept as first parameter the DICTIONARY_ITEM * dictionary dfe now uses internal counter to report; avoided excess variables defined with dfe * dictionary walkthrough callbacks get dictionary acquired items * dictionary reference counters that can be dupped from zero * added advanced functions for get and del * rrdvar managed by dictionaries * thread safety for rrdsetvar * faster rrdvar initialization * rrdvar string lengths should match in all add, del, get functions * rrdvar internals hidden from the rest of the world * rrdvar is now acquired throughout netdata * hide the internal structures of rrdsetvar * rrdsetvar is now acquired through out netdata * rrddimvar managed by dictionary; rrddimvar linked list removed; rrddimvar structures hidden from the rest of netdata * better error handling * dont create variables if not initialized for health * dont create variables if not initialized for health again * rrdfamily is now managed by dictionaries; references of it are acquired dictionary items * type checking on acquired objects * rrdcalc renaming of functions * type checking for rrdfamily_acquired * rrdcalc managed by dictionaries * rrdcalc double free fix * host rrdvars is always needed * attempt to fix deadlock 1 * attempt to fix deadlock 2 * Remove unused variable * attempt to fix deadlock 3 * snprintfz * rrdcalc index in rrdset fix * Stop storing active charts and computing chart hashes * Remove store active chart function * Remove compute chart hash function * Remove sql_store_chart_hash function * Remove store_active_dimension function * dictionary delayed destruction * formatting and cleanup * zero dictionary base on rrdsetvar * added internal error to log delayed destructions of dictionaries * typo in rrddimvar * added debugging info to dictionary * debug info * fix for rrdcalc keys being empty * remove forgotten unlock * remove deadlock * Switch to metadata version 5 and drop chart_hash chart_hash_map chart_active dimension_active v_chart_hash * SQL cosmetic changes * do not busy wait while destroying a referenced dictionary * remove deadlock * code cleanup; re-organization; * fast cleanup and flushing of dictionaries * number formatting fixes * do not delete configured alerts when archiving a chart * rrddim obsolete linked list management outside dictionaries * removed duplicate contexts call * fix crash when rrdfamily is not initialized * dont keep rrddimvar referenced * properly cleanup rrdvar * removed some locks * Do not attempt to cleanup chart_hash / chart_hash_map * rrdcalctemplate managed by dictionary * register callbacks on the right dictionary * removed some more locks * rrdcalc secondary index replaced with linked-list; rrdcalc labels updates are now executed by health thread * when looking up for an alarm look using both chart id and chart name * host initialization a bit more modular * init rrdlabels on host update * preparation for dictionary views * improved comment * unused variables without internal checks * service threads isolation and worker info * more worker info in service thread * thread cancelability debugging with internal checks * strings data races addressed; fixes https://github.com/netdata/netdata/issues/13647 * dictionary modularization * Remove unused SQL statement definition * unit-tested thread safety of dictionaries; removed data race conditions on dictionaries and strings; dictionaries now can detect if the caller is holds a write lock and automatically all the calls become their unsafe versions; all direct calls to unsafe version is eliminated * remove worker_is_idle() from the exit of service functions, because we lose the lock time between loops * rewritten dictionary to have 2 separate locks, one for indexing and another for traversal * Update collectors/cgroups.plugin/sys_fs_cgroup.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * Update collectors/cgroups.plugin/sys_fs_cgroup.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * Update collectors/proc.plugin/proc_net_dev.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * fix memory leak in rrdset cache_dir * minor dictionary changes * dont use index locks in single threaded * obsolete dict option * rrddim options and flags separation; rrdset_done() optimization to keep array of reference pointers to rrddim; * fix jump on uninitialized value in dictionary; remove double free of cache_dir * addressed codacy findings * removed debugging code * use the private refcount on dictionaries * make dictionary item desctructors work on dictionary destruction; strictier control on dictionary API; proper cleanup sequence on rrddim; * more dictionary statistics * global statistics about dictionary operations, memory, items, callbacks * dictionary support for views - missing the public API * removed warning about unused parameter * chart and context name for cloud * chart and context name for cloud, again * dictionary statistics fixed; first implementation of dictionary views - not currently used * only the master can globally delete an item * context needs netdata prefix * fix context and chart it of spins * fix for host variables when health is not enabled * run garbage collector on item insert too * Fix info message; remove extra "using" * update dict unittest for new placement of garbage collector * we need RRDHOST->rrdvars for maintaining custom host variables * Health initialization needs the host->host_uuid * split STRING to its own files; no code changes other than that * initialize health unconditionally * unit tests do not pollute the global scope with their variables * Skip initialization when creating archived hosts on startup. When a child connects it will initialize properly Co-authored-by: Stelios Fragkakis <52996999+stelfrag@users.noreply.github.com> Co-authored-by: Vladimir Kobal <vlad@prokk.net>
317 lines
10 KiB
C
317 lines
10 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#include "rrd.h"
|
|
|
|
// the variables as stored in the variables indexes
|
|
// there are 3 indexes:
|
|
// 1. at each chart (RRDSET.rrdvar_root_index)
|
|
// 2. at each context (RRDFAMILY.rrdvar_root_index)
|
|
// 3. at each host (RRDHOST.rrdvar_root_index)
|
|
typedef struct rrdvar {
|
|
STRING *name;
|
|
void *value;
|
|
RRDVAR_FLAGS flags:24;
|
|
RRDVAR_TYPE type:8;
|
|
} RRDVAR;
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// RRDVAR management
|
|
|
|
inline int rrdvar_fix_name(char *variable) {
|
|
int fixed = 0;
|
|
while(*variable) {
|
|
if (!isalnum(*variable) && *variable != '.' && *variable != '_') {
|
|
*variable++ = '_';
|
|
fixed++;
|
|
}
|
|
else
|
|
variable++;
|
|
}
|
|
|
|
return fixed;
|
|
}
|
|
|
|
inline STRING *rrdvar_name_to_string(const char *name) {
|
|
char *variable = strdupz(name);
|
|
rrdvar_fix_name(variable);
|
|
STRING *name_string = string_strdupz(variable);
|
|
freez(variable);
|
|
return name_string;
|
|
}
|
|
|
|
struct rrdvar_constructor {
|
|
STRING *name;
|
|
void *value;
|
|
RRDVAR_FLAGS options:16;
|
|
RRDVAR_TYPE type:8;
|
|
|
|
enum {
|
|
RRDVAR_REACT_NONE = 0,
|
|
RRDVAR_REACT_NEW = (1 << 0),
|
|
} react_action;
|
|
};
|
|
|
|
static void rrdvar_insert_callback(const DICTIONARY_ITEM *item __maybe_unused, void *rrdvar, void *constructor_data) {
|
|
RRDVAR *rv = rrdvar;
|
|
struct rrdvar_constructor *ctr = constructor_data;
|
|
|
|
ctr->options &= ~RRDVAR_OPTIONS_REMOVED_ON_NEW_OBJECTS;
|
|
|
|
rv->name = string_dup(ctr->name);
|
|
rv->type = ctr->type;
|
|
rv->flags = ctr->options;
|
|
|
|
if(!ctr->value) {
|
|
NETDATA_DOUBLE *v = mallocz(sizeof(NETDATA_DOUBLE));
|
|
*v = NAN;
|
|
rv->value = v;
|
|
rv->flags |= RRDVAR_FLAG_ALLOCATED;
|
|
}
|
|
else
|
|
rv->value = ctr->value;
|
|
|
|
ctr->react_action = RRDVAR_REACT_NEW;
|
|
}
|
|
|
|
static void rrdvar_delete_callback(const DICTIONARY_ITEM *item __maybe_unused, void *rrdvar, void *nothing __maybe_unused) {
|
|
RRDVAR *rv = rrdvar;
|
|
|
|
if(rv->flags & RRDVAR_FLAG_ALLOCATED)
|
|
freez(rv->value);
|
|
|
|
string_freez(rv->name);
|
|
rv->name = NULL;
|
|
}
|
|
|
|
DICTIONARY *rrdvariables_create(void) {
|
|
DICTIONARY *dict = dictionary_create(DICT_OPTION_DONT_OVERWRITE_VALUE);
|
|
|
|
dictionary_register_insert_callback(dict, rrdvar_insert_callback, NULL);
|
|
dictionary_register_delete_callback(dict, rrdvar_delete_callback, NULL);
|
|
|
|
return dict;
|
|
}
|
|
|
|
void rrdvariables_destroy(DICTIONARY *dict) {
|
|
dictionary_destroy(dict);
|
|
}
|
|
|
|
static inline const RRDVAR_ACQUIRED *rrdvar_get_and_acquire(DICTIONARY *dict, STRING *name) {
|
|
return (const RRDVAR_ACQUIRED *)dictionary_get_and_acquire_item_advanced(dict, string2str(name), (ssize_t)string_strlen(name) + 1);
|
|
}
|
|
|
|
inline void rrdvar_release_and_del(DICTIONARY *dict, const RRDVAR_ACQUIRED *rva) {
|
|
if(unlikely(!dict || !rva)) return;
|
|
|
|
RRDVAR *rv = dictionary_acquired_item_value((const DICTIONARY_ITEM *)rva);
|
|
|
|
dictionary_del_advanced(dict, string2str(rv->name), (ssize_t)string_strlen(rv->name) + 1);
|
|
|
|
dictionary_acquired_item_release(dict, (const DICTIONARY_ITEM *)rva);
|
|
}
|
|
|
|
inline const RRDVAR_ACQUIRED *rrdvar_add_and_acquire(const char *scope __maybe_unused, DICTIONARY *dict, STRING *name, RRDVAR_TYPE type, RRDVAR_FLAGS options, void *value) {
|
|
if(unlikely(!dict || !name)) return NULL;
|
|
|
|
struct rrdvar_constructor tmp = {
|
|
.name = name,
|
|
.value = value,
|
|
.type = type,
|
|
.options = options,
|
|
.react_action = RRDVAR_REACT_NONE,
|
|
};
|
|
return (const RRDVAR_ACQUIRED *)dictionary_set_and_acquire_item_advanced(dict, string2str(name), (ssize_t)string_strlen(name) + 1, NULL, sizeof(RRDVAR), &tmp);
|
|
}
|
|
|
|
void rrdvar_delete_all(DICTIONARY *dict) {
|
|
dictionary_flush(dict);
|
|
}
|
|
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// CUSTOM HOST VARIABLES
|
|
|
|
inline int rrdvar_walkthrough_read(DICTIONARY *dict, int (*callback)(const DICTIONARY_ITEM *item, void *rrdvar, void *data), void *data) {
|
|
if(unlikely(!dict)) return 0; // when health is not enabled
|
|
return dictionary_walkthrough_read(dict, callback, data);
|
|
}
|
|
|
|
const RRDVAR_ACQUIRED *rrdvar_custom_host_variable_add_and_acquire(RRDHOST *host, const char *name) {
|
|
DICTIONARY *dict = host->rrdvars;
|
|
if(unlikely(!dict)) return NULL; // when health is not enabled
|
|
|
|
STRING *name_string = rrdvar_name_to_string(name);
|
|
|
|
const RRDVAR_ACQUIRED *rva = rrdvar_add_and_acquire("host", dict, name_string, RRDVAR_TYPE_CALCULATED, RRDVAR_FLAG_CUSTOM_HOST_VAR, NULL);
|
|
|
|
string_freez(name_string);
|
|
return rva;
|
|
}
|
|
|
|
void rrdvar_custom_host_variable_set(RRDHOST *host, const RRDVAR_ACQUIRED *rva, NETDATA_DOUBLE value) {
|
|
if(unlikely(!host->rrdvars || !rva)) return; // when health is not enabled
|
|
|
|
if(rrdvar_type(rva) != RRDVAR_TYPE_CALCULATED || !(rrdvar_flags(rva) & (RRDVAR_FLAG_CUSTOM_HOST_VAR | RRDVAR_FLAG_ALLOCATED)))
|
|
error("requested to set variable '%s' to value " NETDATA_DOUBLE_FORMAT " but the variable is not a custom one.", rrdvar_name(rva), value);
|
|
else {
|
|
RRDVAR *rv = dictionary_acquired_item_value((const DICTIONARY_ITEM *)rva);
|
|
NETDATA_DOUBLE *v = rv->value;
|
|
if(*v != value) {
|
|
*v = value;
|
|
|
|
// if the host is streaming, send this variable upstream immediately
|
|
rrdpush_sender_send_this_host_variable_now(host, rva);
|
|
}
|
|
}
|
|
}
|
|
|
|
void rrdvar_release(DICTIONARY *dict, const RRDVAR_ACQUIRED *rva) {
|
|
if(unlikely(!dict || !rva)) return; // when health is not enabled
|
|
dictionary_acquired_item_release(dict, (const DICTIONARY_ITEM *)rva);
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// RRDVAR lookup
|
|
|
|
NETDATA_DOUBLE rrdvar2number(const RRDVAR_ACQUIRED *rva) {
|
|
if(unlikely(!rva)) return NAN;
|
|
|
|
RRDVAR *rv = dictionary_acquired_item_value((const DICTIONARY_ITEM *)rva);
|
|
|
|
switch(rv->type) {
|
|
case RRDVAR_TYPE_CALCULATED: {
|
|
NETDATA_DOUBLE *n = (NETDATA_DOUBLE *)rv->value;
|
|
return *n;
|
|
}
|
|
|
|
case RRDVAR_TYPE_TIME_T: {
|
|
time_t *n = (time_t *)rv->value;
|
|
return (NETDATA_DOUBLE)*n;
|
|
}
|
|
|
|
case RRDVAR_TYPE_COLLECTED: {
|
|
collected_number *n = (collected_number *)rv->value;
|
|
return (NETDATA_DOUBLE)*n;
|
|
}
|
|
|
|
case RRDVAR_TYPE_TOTAL: {
|
|
total_number *n = (total_number *)rv->value;
|
|
return (NETDATA_DOUBLE)*n;
|
|
}
|
|
|
|
case RRDVAR_TYPE_INT: {
|
|
int *n = (int *)rv->value;
|
|
return *n;
|
|
}
|
|
|
|
default:
|
|
error("I don't know how to convert RRDVAR type %u to NETDATA_DOUBLE", rv->type);
|
|
return NAN;
|
|
}
|
|
}
|
|
|
|
int health_variable_lookup(STRING *variable, RRDCALC *rc, NETDATA_DOUBLE *result) {
|
|
RRDSET *st = rc->rrdset;
|
|
if(!st) return 0;
|
|
|
|
RRDHOST *host = st->rrdhost;
|
|
const RRDVAR_ACQUIRED *rva;
|
|
|
|
rva = rrdvar_get_and_acquire(st->rrdvars, variable);
|
|
if(rva) {
|
|
*result = rrdvar2number(rva);
|
|
dictionary_acquired_item_release(st->rrdvars, (const DICTIONARY_ITEM *)rva);
|
|
return 1;
|
|
}
|
|
|
|
rva = rrdvar_get_and_acquire(rrdfamily_rrdvars_dict(st->rrdfamily), variable);
|
|
if(rva) {
|
|
*result = rrdvar2number(rva);
|
|
dictionary_acquired_item_release(rrdfamily_rrdvars_dict(st->rrdfamily), (const DICTIONARY_ITEM *)rva);
|
|
return 1;
|
|
}
|
|
|
|
rva = rrdvar_get_and_acquire(host->rrdvars, variable);
|
|
if(rva) {
|
|
*result = rrdvar2number(rva);
|
|
dictionary_acquired_item_release(host->rrdvars, (const DICTIONARY_ITEM *)rva);
|
|
return 1;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// RRDVAR to JSON
|
|
|
|
struct variable2json_helper {
|
|
BUFFER *buf;
|
|
size_t counter;
|
|
RRDVAR_FLAGS options;
|
|
};
|
|
|
|
static int single_variable2json_callback(const DICTIONARY_ITEM *item __maybe_unused, void *entry __maybe_unused, void *helper_data) {
|
|
struct variable2json_helper *helper = (struct variable2json_helper *)helper_data;
|
|
const RRDVAR_ACQUIRED *rva = (const RRDVAR_ACQUIRED *)item;
|
|
NETDATA_DOUBLE value = rrdvar2number(rva);
|
|
|
|
if (helper->options == RRDVAR_FLAG_NONE || rrdvar_flags(rva) & helper->options) {
|
|
if(unlikely(isnan(value) || isinf(value)))
|
|
buffer_sprintf(helper->buf, "%s\n\t\t\"%s\": null", helper->counter?",":"", rrdvar_name(rva));
|
|
else
|
|
buffer_sprintf(helper->buf, "%s\n\t\t\"%s\": %0.5" NETDATA_DOUBLE_MODIFIER, helper->counter?",":"", rrdvar_name(rva), (NETDATA_DOUBLE)value);
|
|
|
|
helper->counter++;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
void health_api_v1_chart_custom_variables2json(RRDSET *st, BUFFER *buf) {
|
|
struct variable2json_helper helper = {
|
|
.buf = buf,
|
|
.counter = 0,
|
|
.options = RRDVAR_FLAG_CUSTOM_CHART_VAR};
|
|
|
|
buffer_sprintf(buf, "{");
|
|
rrdvar_walkthrough_read(st->rrdvars, single_variable2json_callback, &helper);
|
|
buffer_strcat(buf, "\n\t\t\t}");
|
|
}
|
|
|
|
void health_api_v1_chart_variables2json(RRDSET *st, BUFFER *buf) {
|
|
RRDHOST *host = st->rrdhost;
|
|
|
|
struct variable2json_helper helper = {
|
|
.buf = buf,
|
|
.counter = 0,
|
|
.options = RRDVAR_FLAG_NONE};
|
|
|
|
buffer_sprintf(buf, "{\n\t\"chart\": \"%s\",\n\t\"chart_name\": \"%s\",\n\t\"chart_context\": \"%s\",\n\t\"chart_variables\": {", rrdset_id(st), rrdset_name(st), rrdset_context(st));
|
|
rrdvar_walkthrough_read(st->rrdvars, single_variable2json_callback, &helper);
|
|
|
|
buffer_sprintf(buf, "\n\t},\n\t\"family\": \"%s\",\n\t\"family_variables\": {", rrdset_family(st));
|
|
helper.counter = 0;
|
|
rrdvar_walkthrough_read(rrdfamily_rrdvars_dict(st->rrdfamily), single_variable2json_callback, &helper);
|
|
|
|
buffer_sprintf(buf, "\n\t},\n\t\"host\": \"%s\",\n\t\"host_variables\": {", rrdhost_hostname(host));
|
|
helper.counter = 0;
|
|
rrdvar_walkthrough_read(host->rrdvars, single_variable2json_callback, &helper);
|
|
|
|
buffer_strcat(buf, "\n\t}\n}\n");
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// RRDVAR private members examination
|
|
|
|
const char *rrdvar_name(const RRDVAR_ACQUIRED *rva) {
|
|
return dictionary_acquired_item_name((const DICTIONARY_ITEM *)rva);
|
|
}
|
|
|
|
RRDVAR_FLAGS rrdvar_flags(const RRDVAR_ACQUIRED *rva) {
|
|
RRDVAR *rv = dictionary_acquired_item_value((const DICTIONARY_ITEM *)rva);
|
|
return rv->flags;
|
|
}
|
|
RRDVAR_TYPE rrdvar_type(const RRDVAR_ACQUIRED *rva) {
|
|
RRDVAR *rv = dictionary_acquired_item_value((const DICTIONARY_ITEM *)rva);
|
|
return rv->type;
|
|
}
|