mirror of
https://github.com/netdata/netdata.git
synced 2025-04-06 06:25:32 +00:00
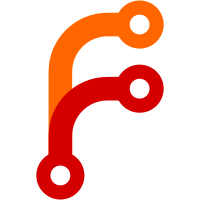
* rrdset - in progress * rrdset optimal constructor; rrdset conflict * rrdset final touches * re-organization of rrdset object members * prevent use-after-free * dictionary dfe supports also counting of iterations * rrddim managed by dictionary * rrd.h cleanup * DICTIONARY_ITEM now is referencing actual dictionary items in the code * removed rrdset linked list * Revert "removed rrdset linked list" This reverts commit 690d6a588b4b99619c2c5e10f84e8f868ae6def5. * removed rrdset linked list * added comments * Switch chart uuid to static allocation in rrdset Remove unused functions * rrdset_archive() and friends... * always create rrdfamily * enable ml_free_dimension * rrddim_foreach done with dfe * most custom rrddim loops replaced with rrddim_foreach * removed accesses to rrddim->dimensions * removed locks that are no longer needed * rrdsetvar is now managed by the dictionary * set rrdset is rrdsetvar, fixes https://github.com/netdata/netdata/pull/13646#issuecomment-1242574853 * conflict callback of rrdsetvar now properly checks if it has to reset the variable * dictionary registered callbacks accept as first parameter the DICTIONARY_ITEM * dictionary dfe now uses internal counter to report; avoided excess variables defined with dfe * dictionary walkthrough callbacks get dictionary acquired items * dictionary reference counters that can be dupped from zero * added advanced functions for get and del * rrdvar managed by dictionaries * thread safety for rrdsetvar * faster rrdvar initialization * rrdvar string lengths should match in all add, del, get functions * rrdvar internals hidden from the rest of the world * rrdvar is now acquired throughout netdata * hide the internal structures of rrdsetvar * rrdsetvar is now acquired through out netdata * rrddimvar managed by dictionary; rrddimvar linked list removed; rrddimvar structures hidden from the rest of netdata * better error handling * dont create variables if not initialized for health * dont create variables if not initialized for health again * rrdfamily is now managed by dictionaries; references of it are acquired dictionary items * type checking on acquired objects * rrdcalc renaming of functions * type checking for rrdfamily_acquired * rrdcalc managed by dictionaries * rrdcalc double free fix * host rrdvars is always needed * attempt to fix deadlock 1 * attempt to fix deadlock 2 * Remove unused variable * attempt to fix deadlock 3 * snprintfz * rrdcalc index in rrdset fix * Stop storing active charts and computing chart hashes * Remove store active chart function * Remove compute chart hash function * Remove sql_store_chart_hash function * Remove store_active_dimension function * dictionary delayed destruction * formatting and cleanup * zero dictionary base on rrdsetvar * added internal error to log delayed destructions of dictionaries * typo in rrddimvar * added debugging info to dictionary * debug info * fix for rrdcalc keys being empty * remove forgotten unlock * remove deadlock * Switch to metadata version 5 and drop chart_hash chart_hash_map chart_active dimension_active v_chart_hash * SQL cosmetic changes * do not busy wait while destroying a referenced dictionary * remove deadlock * code cleanup; re-organization; * fast cleanup and flushing of dictionaries * number formatting fixes * do not delete configured alerts when archiving a chart * rrddim obsolete linked list management outside dictionaries * removed duplicate contexts call * fix crash when rrdfamily is not initialized * dont keep rrddimvar referenced * properly cleanup rrdvar * removed some locks * Do not attempt to cleanup chart_hash / chart_hash_map * rrdcalctemplate managed by dictionary * register callbacks on the right dictionary * removed some more locks * rrdcalc secondary index replaced with linked-list; rrdcalc labels updates are now executed by health thread * when looking up for an alarm look using both chart id and chart name * host initialization a bit more modular * init rrdlabels on host update * preparation for dictionary views * improved comment * unused variables without internal checks * service threads isolation and worker info * more worker info in service thread * thread cancelability debugging with internal checks * strings data races addressed; fixes https://github.com/netdata/netdata/issues/13647 * dictionary modularization * Remove unused SQL statement definition * unit-tested thread safety of dictionaries; removed data race conditions on dictionaries and strings; dictionaries now can detect if the caller is holds a write lock and automatically all the calls become their unsafe versions; all direct calls to unsafe version is eliminated * remove worker_is_idle() from the exit of service functions, because we lose the lock time between loops * rewritten dictionary to have 2 separate locks, one for indexing and another for traversal * Update collectors/cgroups.plugin/sys_fs_cgroup.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * Update collectors/cgroups.plugin/sys_fs_cgroup.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * Update collectors/proc.plugin/proc_net_dev.c Co-authored-by: Vladimir Kobal <vlad@prokk.net> * fix memory leak in rrdset cache_dir * minor dictionary changes * dont use index locks in single threaded * obsolete dict option * rrddim options and flags separation; rrdset_done() optimization to keep array of reference pointers to rrddim; * fix jump on uninitialized value in dictionary; remove double free of cache_dir * addressed codacy findings * removed debugging code * use the private refcount on dictionaries * make dictionary item desctructors work on dictionary destruction; strictier control on dictionary API; proper cleanup sequence on rrddim; * more dictionary statistics * global statistics about dictionary operations, memory, items, callbacks * dictionary support for views - missing the public API * removed warning about unused parameter * chart and context name for cloud * chart and context name for cloud, again * dictionary statistics fixed; first implementation of dictionary views - not currently used * only the master can globally delete an item * context needs netdata prefix * fix context and chart it of spins * fix for host variables when health is not enabled * run garbage collector on item insert too * Fix info message; remove extra "using" * update dict unittest for new placement of garbage collector * we need RRDHOST->rrdvars for maintaining custom host variables * Health initialization needs the host->host_uuid * split STRING to its own files; no code changes other than that * initialize health unconditionally * unit tests do not pollute the global scope with their variables * Skip initialization when creating archived hosts on startup. When a child connects it will initialize properly Co-authored-by: Stelios Fragkakis <52996999+stelfrag@users.noreply.github.com> Co-authored-by: Vladimir Kobal <vlad@prokk.net>
147 lines
5.8 KiB
C
147 lines
5.8 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#include "daemon/common.h"
|
|
#include "registry_internals.h"
|
|
|
|
int registry_init(void) {
|
|
char filename[FILENAME_MAX + 1];
|
|
|
|
// registry enabled?
|
|
if(web_server_mode != WEB_SERVER_MODE_NONE) {
|
|
registry.enabled = config_get_boolean(CONFIG_SECTION_REGISTRY, "enabled", 0);
|
|
}
|
|
else {
|
|
info("Registry is disabled - use the central netdata");
|
|
config_set_boolean(CONFIG_SECTION_REGISTRY, "enabled", 0);
|
|
registry.enabled = 0;
|
|
}
|
|
|
|
// pathnames
|
|
snprintfz(filename, FILENAME_MAX, "%s/registry", netdata_configured_varlib_dir);
|
|
registry.pathname = config_get(CONFIG_SECTION_DIRECTORIES, "registry", filename);
|
|
if(mkdir(registry.pathname, 0770) == -1 && errno != EEXIST)
|
|
fatal("Cannot create directory '%s'.", registry.pathname);
|
|
|
|
// filenames
|
|
snprintfz(filename, FILENAME_MAX, "%s/netdata.public.unique.id", registry.pathname);
|
|
registry.machine_guid_filename = config_get(CONFIG_SECTION_REGISTRY, "netdata unique id file", filename);
|
|
|
|
snprintfz(filename, FILENAME_MAX, "%s/registry.db", registry.pathname);
|
|
registry.db_filename = config_get(CONFIG_SECTION_REGISTRY, "registry db file", filename);
|
|
|
|
snprintfz(filename, FILENAME_MAX, "%s/registry-log.db", registry.pathname);
|
|
registry.log_filename = config_get(CONFIG_SECTION_REGISTRY, "registry log file", filename);
|
|
|
|
// configuration options
|
|
registry.save_registry_every_entries = (unsigned long long)config_get_number(CONFIG_SECTION_REGISTRY, "registry save db every new entries", 1000000);
|
|
registry.persons_expiration = config_get_number(CONFIG_SECTION_REGISTRY, "registry expire idle persons days", 365) * 86400;
|
|
registry.registry_domain = config_get(CONFIG_SECTION_REGISTRY, "registry domain", "");
|
|
registry.registry_to_announce = config_get(CONFIG_SECTION_REGISTRY, "registry to announce", "https://registry.my-netdata.io");
|
|
registry.hostname = config_get(CONFIG_SECTION_REGISTRY, "registry hostname", netdata_configured_hostname);
|
|
registry.verify_cookies_redirects = config_get_boolean(CONFIG_SECTION_REGISTRY, "verify browser cookies support", 1);
|
|
registry.enable_cookies_samesite_secure = config_get_boolean(CONFIG_SECTION_REGISTRY, "enable cookies SameSite and Secure", 1);
|
|
|
|
registry_update_cloud_base_url();
|
|
setenv("NETDATA_REGISTRY_HOSTNAME", registry.hostname, 1);
|
|
setenv("NETDATA_REGISTRY_URL", registry.registry_to_announce, 1);
|
|
|
|
registry.max_url_length = (size_t)config_get_number(CONFIG_SECTION_REGISTRY, "max URL length", 1024);
|
|
if(registry.max_url_length < 10) {
|
|
registry.max_url_length = 10;
|
|
config_set_number(CONFIG_SECTION_REGISTRY, "max URL length", (long long)registry.max_url_length);
|
|
}
|
|
|
|
registry.max_name_length = (size_t)config_get_number(CONFIG_SECTION_REGISTRY, "max URL name length", 50);
|
|
if(registry.max_name_length < 10) {
|
|
registry.max_name_length = 10;
|
|
config_set_number(CONFIG_SECTION_REGISTRY, "max URL name length", (long long)registry.max_name_length);
|
|
}
|
|
|
|
// initialize entries counters
|
|
registry.persons_count = 0;
|
|
registry.machines_count = 0;
|
|
registry.usages_count = 0;
|
|
registry.urls_count = 0;
|
|
registry.persons_urls_count = 0;
|
|
registry.machines_urls_count = 0;
|
|
|
|
// initialize memory counters
|
|
registry.persons_memory = 0;
|
|
registry.machines_memory = 0;
|
|
registry.urls_memory = 0;
|
|
registry.persons_urls_memory = 0;
|
|
registry.machines_urls_memory = 0;
|
|
|
|
// initialize locks
|
|
netdata_mutex_init(®istry.lock);
|
|
|
|
// create dictionaries
|
|
registry.persons = dictionary_create(REGISTRY_DICTIONARY_OPTIONS);
|
|
registry.machines = dictionary_create(REGISTRY_DICTIONARY_OPTIONS);
|
|
avl_init(®istry.registry_urls_root_index, registry_url_compare);
|
|
|
|
// load the registry database
|
|
if(registry.enabled) {
|
|
registry_log_open();
|
|
registry_db_load();
|
|
registry_log_load();
|
|
|
|
if(unlikely(registry_db_should_be_saved()))
|
|
registry_db_save();
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int machine_urls_delete_callback(const DICTIONARY_ITEM *item __maybe_unused, void *entry, void *data) {
|
|
REGISTRY_MACHINE *m = (REGISTRY_MACHINE *)data;
|
|
(void)m;
|
|
|
|
REGISTRY_MACHINE_URL *mu = (REGISTRY_MACHINE_URL *)entry;
|
|
|
|
debug(D_REGISTRY, "Registry: unlinking url '%s' from machine", mu->url->url);
|
|
registry_url_unlink(mu->url);
|
|
|
|
debug(D_REGISTRY, "Registry: freeing machine url");
|
|
freez(mu);
|
|
|
|
return 1;
|
|
}
|
|
|
|
static int machine_delete_callback(const DICTIONARY_ITEM *item __maybe_unused, void *entry, void *data __maybe_unused) {
|
|
REGISTRY_MACHINE *m = (REGISTRY_MACHINE *)entry;
|
|
int ret = dictionary_walkthrough_read(m->machine_urls, machine_urls_delete_callback, m);
|
|
|
|
dictionary_destroy(m->machine_urls);
|
|
freez(m);
|
|
|
|
return ret + 1;
|
|
}
|
|
static int registry_person_del_callback(const DICTIONARY_ITEM *item __maybe_unused, void *entry, void *d __maybe_unused) {
|
|
REGISTRY_PERSON *p = (REGISTRY_PERSON *)entry;
|
|
|
|
debug(D_REGISTRY, "Registry: registry_person_del('%s'): deleting person", p->guid);
|
|
|
|
while(p->person_urls.root)
|
|
registry_person_unlink_from_url(p, (REGISTRY_PERSON_URL *)p->person_urls.root);
|
|
|
|
//debug(D_REGISTRY, "Registry: deleting person '%s' from persons registry", p->guid);
|
|
//dictionary_del(registry.persons, p->guid);
|
|
|
|
debug(D_REGISTRY, "Registry: freeing person '%s'", p->guid);
|
|
freez(p);
|
|
|
|
return 1;
|
|
}
|
|
|
|
void registry_free(void) {
|
|
if(!registry.enabled) return;
|
|
|
|
debug(D_REGISTRY, "Registry: destroying persons dictionary");
|
|
dictionary_walkthrough_read(registry.persons, registry_person_del_callback, NULL);
|
|
dictionary_destroy(registry.persons);
|
|
|
|
debug(D_REGISTRY, "Registry: destroying machines dictionary");
|
|
dictionary_walkthrough_read(registry.machines, machine_delete_callback, NULL);
|
|
dictionary_destroy(registry.machines);
|
|
}
|