mirror of
https://github.com/netdata/netdata.git
synced 2025-05-01 16:09:52 +00:00
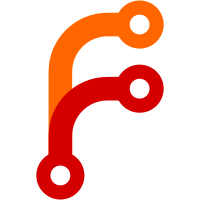
* expose the order of group by * key renames in json wrapper v2 * added group by context and group by units * added view_average_values * fix for view_average_values when percentage is specified * option group-by-labels is enabling the exposure of all the labels that are used for each of the final grouped dimensions * when executing group by queries, allocate one dimension data at a time - not all of them * respect hidden dimensions * cancel running data query on socket error * use poll to detect socket errors * use POLLRDHUP to detect half closed connections * make sure POLLRDHUP is available * do not destroy aral-by-size arals * completed documentation of /api/v2/data. * moved min, max back to view; updated swagger yaml and json * default format for /api/v2/data is json2
64 lines
No EOL
2.4 KiB
C
64 lines
No EOL
2.4 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
#include "web_api.h"
|
|
|
|
int web_client_api_request_vX(RRDHOST *host, struct web_client *w, char *url, struct web_api_command *api_commands) {
|
|
if(unlikely(!url || !*url)) {
|
|
buffer_flush(w->response.data);
|
|
buffer_sprintf(w->response.data, "Which API command?");
|
|
return HTTP_RESP_BAD_REQUEST;
|
|
}
|
|
|
|
uint32_t hash = simple_hash(url);
|
|
|
|
for(int i = 0; api_commands[i].command ; i++) {
|
|
if(unlikely(hash == api_commands[i].hash && !strcmp(url, api_commands[i].command))) {
|
|
if(unlikely(api_commands[i].acl != WEB_CLIENT_ACL_NOCHECK) && !(w->acl & api_commands[i].acl))
|
|
return web_client_permission_denied(w);
|
|
|
|
return api_commands[i].callback(host, w, (w->decoded_query_string + 1));
|
|
}
|
|
}
|
|
|
|
buffer_flush(w->response.data);
|
|
buffer_strcat(w->response.data, "Unsupported API command: ");
|
|
buffer_strcat_htmlescape(w->response.data, url);
|
|
return HTTP_RESP_NOT_FOUND;
|
|
}
|
|
|
|
RRDCONTEXT_TO_JSON_OPTIONS rrdcontext_to_json_parse_options(char *o) {
|
|
RRDCONTEXT_TO_JSON_OPTIONS options = RRDCONTEXT_OPTION_NONE;
|
|
char *tok;
|
|
|
|
while(o && *o && (tok = mystrsep(&o, ", |"))) {
|
|
if(!*tok) continue;
|
|
|
|
if(!strcmp(tok, "full") || !strcmp(tok, "all"))
|
|
options |= RRDCONTEXT_OPTIONS_ALL;
|
|
else if(!strcmp(tok, "charts") || !strcmp(tok, "instances"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_INSTANCES;
|
|
else if(!strcmp(tok, "dimensions") || !strcmp(tok, "metrics"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_METRICS;
|
|
else if(!strcmp(tok, "queue"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_QUEUED;
|
|
else if(!strcmp(tok, "flags"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_FLAGS;
|
|
else if(!strcmp(tok, "uuids"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_UUIDS;
|
|
else if(!strcmp(tok, "deleted"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_DELETED;
|
|
else if(!strcmp(tok, "labels"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_LABELS;
|
|
else if(!strcmp(tok, "deepscan"))
|
|
options |= RRDCONTEXT_OPTION_DEEPSCAN;
|
|
else if(!strcmp(tok, "hidden"))
|
|
options |= RRDCONTEXT_OPTION_SHOW_HIDDEN;
|
|
}
|
|
|
|
return options;
|
|
}
|
|
|
|
bool web_client_interrupt_callback(void *data) {
|
|
struct web_client *w = data;
|
|
return sock_has_output_error(w->ofd);
|
|
} |