mirror of
https://github.com/nextcloud/server.git
synced 2024-12-29 16:38:28 +00:00
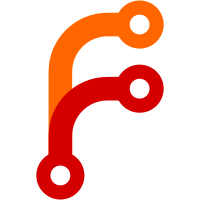
Previously declarative settings were sorted by priority but behind the "native" settings, this is now fixed, meaning a declarative setting with higher priority than an `ISetting` will be correctly rendered before that `ISetting` in the settings list. Signed-off-by: Ferdinand Thiessen <opensource@fthiessen.de>
59 lines
1.6 KiB
PHP
59 lines
1.6 KiB
PHP
<?php
|
|
/**
|
|
* SPDX-FileCopyrightText: 2017 Nextcloud GmbH and Nextcloud contributors
|
|
* SPDX-License-Identifier: AGPL-3.0-or-later
|
|
*/
|
|
namespace OCA\Settings\Controller;
|
|
|
|
use OCP\AppFramework\Controller;
|
|
use OCP\AppFramework\Http\Attribute\NoAdminRequired;
|
|
use OCP\AppFramework\Http\Attribute\NoCSRFRequired;
|
|
use OCP\AppFramework\Http\Attribute\OpenAPI;
|
|
use OCP\AppFramework\Http\TemplateResponse;
|
|
use OCP\AppFramework\Services\IInitialState;
|
|
use OCP\Group\ISubAdmin;
|
|
use OCP\IGroupManager;
|
|
use OCP\INavigationManager;
|
|
use OCP\IRequest;
|
|
use OCP\IUserSession;
|
|
use OCP\Settings\IDeclarativeManager;
|
|
use OCP\Settings\IManager as ISettingsManager;
|
|
|
|
#[OpenAPI(scope: OpenAPI::SCOPE_IGNORE)]
|
|
class PersonalSettingsController extends Controller {
|
|
use CommonSettingsTrait;
|
|
|
|
public function __construct(
|
|
$appName,
|
|
IRequest $request,
|
|
INavigationManager $navigationManager,
|
|
ISettingsManager $settingsManager,
|
|
IUserSession $userSession,
|
|
IGroupManager $groupManager,
|
|
ISubAdmin $subAdmin,
|
|
IDeclarativeManager $declarativeSettingsManager,
|
|
IInitialState $initialState,
|
|
) {
|
|
parent::__construct($appName, $request);
|
|
$this->navigationManager = $navigationManager;
|
|
$this->settingsManager = $settingsManager;
|
|
$this->userSession = $userSession;
|
|
$this->subAdmin = $subAdmin;
|
|
$this->groupManager = $groupManager;
|
|
$this->declarativeSettingsManager = $declarativeSettingsManager;
|
|
$this->initialState = $initialState;
|
|
}
|
|
|
|
/**
|
|
* @NoSubAdminRequired
|
|
*/
|
|
#[NoAdminRequired]
|
|
#[NoCSRFRequired]
|
|
public function index(string $section): TemplateResponse {
|
|
return $this->getIndexResponse(
|
|
'personal',
|
|
$section,
|
|
);
|
|
}
|
|
}
|