mirror of
https://github.com/nextcloud/server.git
synced 2024-12-29 16:38:28 +00:00
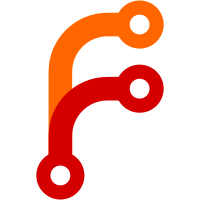
This is required to not break behavior on zip download (apps should be able to react to zip download especially for shares). Signed-off-by: Ferdinand Thiessen <opensource@fthiessen.de>
99 lines
1.9 KiB
PHP
99 lines
1.9 KiB
PHP
<?php
|
|
|
|
declare(strict_types=1);
|
|
|
|
/**
|
|
* SPDX-FileCopyrightText: 2022 Nextcloud GmbH and Nextcloud contributors
|
|
* SPDX-License-Identifier: AGPL-3.0-or-later
|
|
*/
|
|
|
|
namespace OCP\Files\Events;
|
|
|
|
use OCP\EventDispatcher\Event;
|
|
use OCP\Files\Folder;
|
|
|
|
/**
|
|
* This event is triggered before a archive is created when a user requested
|
|
* downloading a folder or multiple files.
|
|
*
|
|
* By setting `successful` to false the tar creation can be aborted and the download denied.
|
|
*
|
|
* @since 25.0.0
|
|
*/
|
|
class BeforeZipCreatedEvent extends Event {
|
|
private string $directory;
|
|
private bool $successful = true;
|
|
private ?string $errorMessage = null;
|
|
private ?Folder $folder = null;
|
|
|
|
/**
|
|
* @param list<string> $files
|
|
* @since 25.0.0
|
|
* @since 31.0.0 support `OCP\Files\Folder` as `$directory` parameter - passing a string is deprecated now
|
|
*/
|
|
public function __construct(
|
|
string|Folder $directory,
|
|
private array $files,
|
|
) {
|
|
parent::__construct();
|
|
if ($directory instanceof Folder) {
|
|
$this->directory = $directory->getPath();
|
|
$this->folder = $directory;
|
|
} else {
|
|
$this->directory = $directory;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @since 31.0.0
|
|
*/
|
|
public function getFolder(): ?Folder {
|
|
return $this->folder;
|
|
}
|
|
|
|
/**
|
|
* @since 25.0.0
|
|
*/
|
|
public function getDirectory(): string {
|
|
return $this->directory;
|
|
}
|
|
|
|
/**
|
|
* @since 25.0.0
|
|
*/
|
|
public function getFiles(): array {
|
|
return $this->files;
|
|
}
|
|
|
|
/**
|
|
* @since 25.0.0
|
|
*/
|
|
public function isSuccessful(): bool {
|
|
return $this->successful;
|
|
}
|
|
|
|
/**
|
|
* Set if the event was successful
|
|
*
|
|
* @since 25.0.0
|
|
*/
|
|
public function setSuccessful(bool $successful): void {
|
|
$this->successful = $successful;
|
|
}
|
|
|
|
/**
|
|
* Get the error message, if any
|
|
* @since 25.0.0
|
|
*/
|
|
public function getErrorMessage(): ?string {
|
|
return $this->errorMessage;
|
|
}
|
|
|
|
/**
|
|
* @since 25.0.0
|
|
*/
|
|
public function setErrorMessage(string $errorMessage): void {
|
|
$this->errorMessage = $errorMessage;
|
|
}
|
|
}
|