mirror of
https://github.com/renovatebot/renovate.git
synced 2025-02-28 18:44:03 +00:00
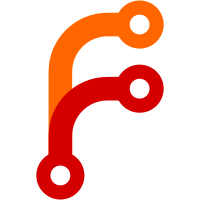
Co-authored-by: Nicolas Bender <nicolas.bender@sap.com> Co-authored-by: HonkingGoose <34918129+HonkingGoose@users.noreply.github.com> Co-authored-by: Michael Kriese <michael.kriese@visualon.de> Co-authored-by: Pavel Busko <pavel.busko@sap.com> Co-authored-by: Johannes Dillmann <j.dillmann@sap.com> Co-authored-by: Michael Kriese <michael.kriese@gmx.de>
66 lines
2.2 KiB
TypeScript
66 lines
2.2 KiB
TypeScript
import { getPkgReleases } from '..';
|
|
import * as httpMock from '../../../../test/http-mock';
|
|
import { BuildpacksRegistryDatasource } from '.';
|
|
|
|
const baseUrl = 'https://registry.buildpacks.io/api/v1/buildpacks/';
|
|
|
|
describe('modules/datasource/buildpacks-registry/index', () => {
|
|
describe('getReleases', () => {
|
|
it('processes real data', async () => {
|
|
httpMock
|
|
.scope(baseUrl)
|
|
.get('/heroku/python')
|
|
.reply(200, {
|
|
latest: {
|
|
version: '0.17.1',
|
|
namespace: 'heroku',
|
|
name: 'python',
|
|
description: "Heroku's buildpack for Python applications.",
|
|
homepage: 'https://github.com/heroku/buildpacks-python',
|
|
licenses: ['BSD-3-Clause'],
|
|
stacks: ['*'],
|
|
id: '75946bf8-3f6a-4af0-a757-614bebfdfcd6',
|
|
},
|
|
versions: [
|
|
{
|
|
version: '0.17.1',
|
|
_link:
|
|
'https://registry.buildpacks.io//api/v1/buildpacks/heroku/python/0.17.1',
|
|
},
|
|
{
|
|
version: '0.17.0',
|
|
_link:
|
|
'https://registry.buildpacks.io//api/v1/buildpacks/heroku/python/0.17.0',
|
|
},
|
|
],
|
|
});
|
|
const res = await getPkgReleases({
|
|
datasource: BuildpacksRegistryDatasource.id,
|
|
packageName: 'heroku/python',
|
|
});
|
|
expect(res).toEqual({
|
|
registryUrl: 'https://registry.buildpacks.io',
|
|
releases: [{ version: '0.17.0' }, { version: '0.17.1' }],
|
|
sourceUrl: 'https://github.com/heroku/buildpacks-python',
|
|
});
|
|
});
|
|
|
|
it('returns null on empty result', async () => {
|
|
httpMock.scope(baseUrl).get('/heroku/empty').reply(200, {});
|
|
const res = await getPkgReleases({
|
|
datasource: BuildpacksRegistryDatasource.id,
|
|
packageName: 'heroku/empty',
|
|
});
|
|
expect(res).toBeNull();
|
|
});
|
|
|
|
it('handles not found', async () => {
|
|
httpMock.scope(baseUrl).get('/heroku/notexisting').reply(404);
|
|
const res = await getPkgReleases({
|
|
datasource: BuildpacksRegistryDatasource.id,
|
|
packageName: 'heroku/notexisting',
|
|
});
|
|
expect(res).toBeNull();
|
|
});
|
|
});
|
|
});
|