mirror of
https://github.com/salesagility/SuiteCRM.git
synced 2025-02-21 20:56:08 +00:00
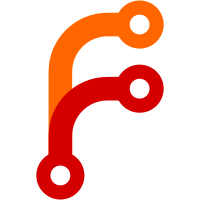
- File: '... /tests/unit/phpunit/modules/Leads/LeadTest.php' - Replaced 1 occurrence(s) of 'new Lead()' - Replaced 1 occurrence(s) of 'new Contact()' - Replaced 1 occurrence(s) of 'new User()' - File: '... /tests/unit/phpunit/modules/AOP_Case_Events/AOP_Case_EventsTest.php' - Replaced 1 occurrence(s) of 'new AOP_Case_Events()' - File: '... /tests/unit/phpunit/modules/AOS_Products/AOS_ProductsTest.php' - Replaced 1 occurrence(s) of 'new User()' - Replaced 3 occurrence(s) of 'new AOS_Products()' - File: '... /tests/unit/phpunit/modules/AOD_Index/AOD_IndexTest.php' - Replaced 10 occurrence(s) of 'new AOD_Index()' - File: '... /tests/unit/phpunit/modules/Contacts/ContactTest.php' - Replaced 16 occurrence(s) of 'new Contact()' - Replaced 1 occurrence(s) of 'new User()' - File: '... /tests/unit/phpunit/modules/SecurityGroups/SecurityGroupTest.php' - Replaced 6 occurrence(s) of 'new Account()' - Replaced 1 occurrence(s) of 'new User()' - Replaced 16 occurrence(s) of 'new SecurityGroup()' - File: '... /tests/unit/phpunit/modules/AM_ProjectTemplates/AM_ProjectTemplatesTest.php' - Replaced 1 occurrence(s) of 'new AM_ProjectTemplates()' - File: '... /tests/unit/phpunit/modules/jjwg_Areas/jjwg_AreasTest.php' - Replaced 15 occurrence(s) of 'new jjwg_Areas()' - File: '... /tests/unit/phpunit/modules/AOR_Charts/AOR_ChartTest.php' - Replaced 1 occurrence(s) of 'new AOR_Report()' - Replaced 8 occurrence(s) of 'new AOR_Chart()' - File: '... /tests/unit/phpunit/modules/Audit/AuditTest.php' - Replaced 2 occurrence(s) of 'new Account()' - Replaced 1 occurrence(s) of 'new User()' - Replaced 10 occurrence(s) of 'new Audit()'
203 lines
8 KiB
PHP
203 lines
8 KiB
PHP
<?php
|
|
|
|
use SuiteCRM\Test\SuitePHPUnitFrameworkTestCase;
|
|
|
|
class AOD_IndexTest extends SuitePHPUnitFrameworkTestCase
|
|
{
|
|
public function testAOD_Index()
|
|
{
|
|
// Execute the constructor and check for the Object type and type attribute
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$this->assertInstanceOf('AOD_Index', $aod_index);
|
|
$this->assertInstanceOf('Basic', $aod_index);
|
|
$this->assertInstanceOf('SugarBean', $aod_index);
|
|
|
|
$this->assertAttributeEquals('AOD_Index', 'module_dir', $aod_index);
|
|
$this->assertAttributeEquals('AOD_Index', 'object_name', $aod_index);
|
|
$this->assertAttributeEquals('aod_index', 'table_name', $aod_index);
|
|
$this->assertAttributeEquals(true, 'new_schema', $aod_index);
|
|
$this->assertAttributeEquals(true, 'disable_row_level_security', $aod_index);
|
|
$this->assertAttributeEquals(false, 'importable', $aod_index);
|
|
$this->assertAttributeEquals(false, 'tracker_visibility', $aod_index);
|
|
}
|
|
|
|
public function testisEnabled()
|
|
{
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
|
|
// execute the method and verify that it returns true
|
|
$result = $aod_index->isEnabled();
|
|
$this->assertTrue($result);
|
|
}
|
|
|
|
public function testfind()
|
|
{
|
|
self::markTestIncomplete('[Zend_Search_Lucene_Exception] File \'modules/AOD_Index/Index/Index/segments_31\' is not readable.');
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
|
|
$aod_index->id = 1;
|
|
$aod_index->location = 'modules/AOD_Index/Index/Index';
|
|
|
|
//execute the method with parameters and verify that it returns true
|
|
$hits = $aod_index->find('/');
|
|
$this->assertTrue(is_array($hits));
|
|
}
|
|
|
|
public function testoptimise()
|
|
{
|
|
self::markTestIncomplete('[Zend_Search_Lucene_Exception] File \'modules/AOD_Index/Index/Index/segments_31\' is not readable.');
|
|
|
|
// test
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$aod_index->id = 1;
|
|
$aod_index->location = 'modules/AOD_Index/Index/Index';
|
|
$last_optimized = $aod_index->last_optimised;
|
|
|
|
//execute the method and test if the last optimized date is changed to a later date/time.
|
|
$aod_index->optimise();
|
|
$this->assertGreaterThan($last_optimized, $aod_index->last_optimised);
|
|
}
|
|
|
|
public function testgetIndex()
|
|
{
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$result = $aod_index->getIndex();
|
|
|
|
//execute the method and verify it returns a different instance of samme type
|
|
$this->assertInstanceOf('AOD_Index', $result);
|
|
$this->assertNotSame($aod_index, $result);
|
|
}
|
|
|
|
public function testgetDocumentForBean()
|
|
{
|
|
$user = new User(1);
|
|
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$result = $aod_index->getDocumentForBean($user);
|
|
|
|
//execute the method and verify that it returns an array
|
|
$this->assertTrue(is_array($result));
|
|
|
|
//verify that returned array has a valid Zend_Search_Lucene_Document instance
|
|
$this->assertInstanceOf('Zend_Search_Lucene_Document', $result['document']);
|
|
}
|
|
|
|
public function testcommit()
|
|
{
|
|
self::markTestIncomplete('File \'modules/AOD_Index/Index/Index/segments_31\' is not readable.');
|
|
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$aod_index->id = 1;
|
|
$aod_index->location = 'modules/AOD_Index/Index/Index';
|
|
|
|
// Execute the method and test that it works and doesn't throw an exception.
|
|
try {
|
|
$aod_index->commit();
|
|
$this->assertTrue(true);
|
|
} catch (Exception $e) {
|
|
$this->fail($e->getMessage() . "\nTrace:\n" . $e->getTraceAsString());
|
|
}
|
|
}
|
|
|
|
public function testisModuleSearchable()
|
|
{
|
|
//test with an invalid module
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('', ''));
|
|
|
|
//test for modules that are searchable
|
|
$this->assertTrue(AOD_Index::isModuleSearchable('DocumentRevisions', 'DocumentRevision'));
|
|
$this->assertTrue(AOD_Index::isModuleSearchable('Cases', 'Case'));
|
|
$this->assertTrue(AOD_Index::isModuleSearchable('Accounts', 'Account'));
|
|
|
|
//test for modules that are not searchable
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('AOD_IndexEvent', 'AOD_IndexEvent'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('AOD_Index', 'AOD_Index'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('AOW_Actions', 'AOW_Action'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('AOW_Conditions', 'AOW_Condition'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('AOW_Processed', 'AOW_Processed'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('SchedulersJobs', 'SchedulersJob'));
|
|
$this->assertFalse(AOD_Index::isModuleSearchable('Users', 'User'));
|
|
}
|
|
|
|
public function testindex()
|
|
{
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$aod_index->id = 1;
|
|
$aod_index->location = 'modules/AOD_Index/Index/Index';
|
|
|
|
//test with a not searchable module, it will return false
|
|
$result = $aod_index->index('Users', 1);
|
|
$this->assertFalse($result);
|
|
|
|
//test with a searchable module but invalid bean id, it will still index it
|
|
$result = $aod_index->index('Accounts', 1);
|
|
$this->assertEquals(null, $result);
|
|
}
|
|
|
|
public function testremove()
|
|
{
|
|
self::markTestIncomplete('File \'modules/AOD_Index/Index/Index/segments_31\' is not readable.');
|
|
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
$aod_index->id = 1;
|
|
$aod_index->location = 'modules/AOD_Index/Index/Index';
|
|
|
|
// Execute the method and test that it works and doesn't throw an exception.
|
|
try {
|
|
$aod_index->remove('Accounts', 1);
|
|
$this->assertTrue(true);
|
|
} catch (Exception $e) {
|
|
$this->fail($e->getMessage() . "\nTrace:\n" . $e->getTraceAsString());
|
|
}
|
|
}
|
|
|
|
public function testgetIndexableModules()
|
|
{
|
|
$expected = array(
|
|
'AM_ProjectTemplates' => 'AM_ProjectTemplates',
|
|
'AM_TaskTemplates' => 'AM_TaskTemplates',
|
|
'AOK_KnowledgeBase' => 'AOK_KnowledgeBase',
|
|
'AOK_Knowledge_Base_Categories' => 'AOK_Knowledge_Base_Categories',
|
|
'AOP_Case_Events' => 'AOP_Case_Events',
|
|
'AOP_Case_Updates' => 'AOP_Case_Updates',
|
|
'AOR_Charts' => 'AOR_Chart',
|
|
'AOR_Conditions' => 'AOR_Condition',
|
|
'AOR_Fields' => 'AOR_Field',
|
|
'AOR_Reports' => 'AOR_Report',
|
|
'AOS_Contracts' => 'AOS_Contracts',
|
|
'AOS_Product_Categories' => 'AOS_Product_Categories',
|
|
'AOW_WorkFlow' => 'AOW_WorkFlow',
|
|
'Accounts' => 'Account',
|
|
'Bugs' => 'Bug',
|
|
'Calls' => 'Call',
|
|
'Calls_Reschedule' => 'Calls_Reschedule',
|
|
'Campaigns' => 'Campaign',
|
|
'Cases' => 'aCase',
|
|
'Contacts' => 'Contact',
|
|
'DocumentRevisions' => 'DocumentRevision',
|
|
'Documents' => 'Document',
|
|
'FP_events' => 'FP_events',
|
|
'Leads' => 'Lead',
|
|
'Meetings' => 'Meeting',
|
|
'Notes' => 'Note',
|
|
'Opportunities' => 'Opportunity',
|
|
'OutboundEmailAccounts' => 'OutboundEmailAccounts',
|
|
'Project' => 'Project',
|
|
'ProjectTask' => 'ProjectTask',
|
|
'ProspectLists' => 'ProspectList',
|
|
'Prospects' => 'Prospect',
|
|
'SurveyQuestionOptions' => 'SurveyQuestionOptions',
|
|
'SurveyQuestionResponses' => 'SurveyQuestionResponses',
|
|
'SurveyQuestions' => 'SurveyQuestions',
|
|
'SurveyResponses' => 'SurveyResponses',
|
|
'Surveys' => 'Surveys',
|
|
'Tasks' => 'Task'
|
|
);
|
|
|
|
$aod_index = BeanFactory::newBean('AOD_Index');
|
|
|
|
//execute the method and verify that it retunrs expected results
|
|
$actual = $aod_index->getIndexableModules();
|
|
$this->assertSame($expected, $actual);
|
|
}
|
|
}
|