mirror of
https://github.com/salesagility/SuiteCRM.git
synced 2025-02-21 20:56:08 +00:00
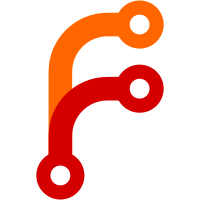
- File: '... /tests/unit/phpunit/modules/CampaignTrackers/CampaignTrackerTest.php' - Replaced 4 occurrence(s) of 'new CampaignTracker()' - Replaced 1 occurrence(s) of 'new User()' - File: '... /tests/unit/phpunit/modules/SugarFeed/SugarFeedTest.php' - Replaced 1 occurrence(s) of 'new Lead()' - Replaced 1 occurrence(s) of 'new User()' - Replaced 1 occurrence(s) of 'new Administration()' - Replaced 5 occurrence(s) of 'new SugarFeed()' - File: '... /tests/unit/phpunit/modules/EmailTemplates/EmailTemplateTest.php' - Replaced 1 occurrence(s) of 'new Lead()' - Replaced 1 occurrence(s) of 'new Prospect()' - Replaced 2 occurrence(s) of 'new Campaign()' - Replaced 2 occurrence(s) of 'new Contact()' - Replaced 2 occurrence(s) of 'new Account()' - Replaced 27 occurrence(s) of 'new EmailTemplate()' - Replaced 2 occurrence(s) of 'new User()' - File: '... /tests/unit/phpunit/modules/EAPM/EAPMTest.php' - Replaced 9 occurrence(s) of 'new EAPM()' - File: '... /tests/unit/phpunit/modules/AOW_Actions/AOW_ActionTest.php' - Replaced 1 occurrence(s) of 'new User()' - Replaced 3 occurrence(s) of 'new AOW_Action()' - Replaced 1 occurrence(s) of 'new AOW_WorkFlow()' - File: '... /tests/unit/phpunit/modules/Emails/EmailTest.php' - Replaced 71 occurrence(s) of 'new Email()' - Replaced 1 occurrence(s) of 'new User()' - Replaced 5 occurrence(s) of 'new InboundEmail()' - File: '... /tests/unit/phpunit/modules/Emails/EmailFromValidatorTest.php' - Replaced 3 occurrence(s) of 'new Email()' - File: '... /tests/unit/phpunit/modules/Emails/NonGmailSentFolderHandlerTest.php' - Replaced 9 occurrence(s) of 'new InboundEmail()' - File: '... /tests/unit/phpunit/modules/Cases/CaseTest.php' - Replaced 15 occurrence(s) of 'new aCase()' - Replaced 1 occurrence(s) of 'new User()' - File: '... /tests/unit/phpunit/modules/OAuthKeys/OAuthKeyTest.php' - Replaced 1 occurrence(s) of 'new User()' - Replaced 4 occurrence(s) of 'new OAuthKey()'
73 lines
2.6 KiB
PHP
73 lines
2.6 KiB
PHP
<?php
|
|
|
|
use SuiteCRM\Test\SuitePHPUnitFrameworkTestCase;
|
|
|
|
class CampaignTrackerTest extends SuitePHPUnitFrameworkTestCase
|
|
{
|
|
public function setUp()
|
|
{
|
|
parent::setUp();
|
|
|
|
global $current_user;
|
|
get_sugar_config_defaults();
|
|
$current_user = BeanFactory::newBean('Users');
|
|
}
|
|
|
|
public function testCampaignTracker()
|
|
{
|
|
// Execute the constructor and check for the Object type and attributes
|
|
$campaignTracker = BeanFactory::newBean('CampaignTrackers');
|
|
$this->assertInstanceOf('CampaignTracker', $campaignTracker);
|
|
$this->assertInstanceOf('SugarBean', $campaignTracker);
|
|
|
|
$this->assertAttributeEquals('CampaignTrackers', 'module_dir', $campaignTracker);
|
|
$this->assertAttributeEquals('CampaignTracker', 'object_name', $campaignTracker);
|
|
$this->assertAttributeEquals('campaign_trkrs', 'table_name', $campaignTracker);
|
|
$this->assertAttributeEquals(true, 'new_schema', $campaignTracker);
|
|
}
|
|
|
|
public function testsave()
|
|
{
|
|
$campaignTracker = BeanFactory::newBean('CampaignTrackers');
|
|
|
|
$campaignTracker->tracker_name = 'test';
|
|
$campaignTracker->is_optout = 1;
|
|
|
|
$campaignTracker->save();
|
|
|
|
//test for record ID to verify that record is saved
|
|
$this->assertTrue(isset($campaignTracker->id));
|
|
$this->assertEquals(36, strlen($campaignTracker->id));
|
|
|
|
//mark the record as deleted and verify that this record cannot be retrieved anymore.
|
|
$campaignTracker->mark_deleted($campaignTracker->id);
|
|
$result = $campaignTracker->retrieve($campaignTracker->id);
|
|
$this->assertEquals(null, $result);
|
|
}
|
|
|
|
public function testget_summary_text()
|
|
{
|
|
$campaignTracker = BeanFactory::newBean('CampaignTrackers');
|
|
|
|
//test without setting name
|
|
$this->assertEquals(null, $campaignTracker->get_summary_text());
|
|
|
|
//test with name set
|
|
$campaignTracker->tracker_name = 'test';
|
|
$this->assertEquals('test', $campaignTracker->get_summary_text());
|
|
}
|
|
|
|
public function testfill_in_additional_detail_fields()
|
|
{
|
|
$campaignTracker = BeanFactory::newBean('CampaignTrackers');
|
|
|
|
//test without is_optout set
|
|
$campaignTracker->fill_in_additional_detail_fields();
|
|
$this->assertStringEndsWith('/index.php?entryPoint=campaign_trackerv2&track=', $campaignTracker->message_url);
|
|
|
|
//test with is_optout set
|
|
$campaignTracker->is_optout = 1;
|
|
$campaignTracker->fill_in_additional_detail_fields();
|
|
$this->assertStringEndsWith('/index.php?entryPoint=removeme&identifier={MESSAGE_ID}', $campaignTracker->message_url);
|
|
}
|
|
}
|